Thi is the code to show svg files in tkinter. You can find also a version that shows png files here. Here, instead, you find the first version of the code with the live coding video and the post with the script, in case you want to see the code from scratch. There is also a version with images into the label widget instead of the canvas.
So, resuming, we have:
- show svg in tkinter (simple script with one image to show)
- first version of slider in tkinter with images in canvas
- first version of slider in tkinter with images in label
The good thing of this version with svg is:
- the images looks better when you change the window size
- the images in svg occupies less space
- you can use inkscape (or other svg apps) to make easily nice slides
- you do not have to convert the files into png every time in inkscape (save time)
- you can easily change the slides from the svg version, rather than from the png
This is the code
import tkinter as tk import glob from PIL import Image, ImageTk import os from svglib.svglib import svg2rlg from reportlab.graphics import renderPM def insertfiles(): "loads the list of files in the directory" for filename in glob.glob("*.svg"): lst.insert(tk.END, filename) def delete_item(event): "Deletes a file in the list: called by lst.bind('<Control-d>', delete_item)" n = lst.curselection() os.remove(lst.get(n)) lst.delete(n) def get_window_size(): "Returns the width and height of the screen to set images and canvas alike it: called by root.bind <Configure>" if root.winfo_width() > 200 and root.winfo_height() >30: w = root.winfo_width() - 200 h = root.winfo_height() - 30 else: w = 200 h = 30 return w, h def showimg(event): "takes the selected image to show it, called by root.bind <Configure> and lst.bind <<ListboxSelect>>" n = lst.curselection() filename = lst.get(n) drawing = svg2rlg(filename) renderPM.drawToFile(drawing, "temp.png", fmt="PNG") im = Image.open("temp.png") im = im.resize((get_window_size()), Image.ANTIALIAS) img = ImageTk.PhotoImage(im) w, h = img.width(), img.height() canvas.image = img canvas.config(width=w, height=h) canvas.create_image(0, 0, image=img, anchor=tk.NW) root.bind("<Configure>", lambda x: showimg(x)) root = tk.Tk() root.geometry("800x600+300+50") lst = tk.Listbox(root, width=20) lst.pack(side="left", fill=tk.BOTH, expand=0) lst.bind("<<ListboxSelect>>", showimg) lst.bind("<Control-d>", delete_item) insertfiles() canvas = tk.Canvas(root) canvas.pack() root.mainloop()
The video explanation of the code
New version without saving the png on the drive
Go here to see the new version, where there are no files saved on the drive.
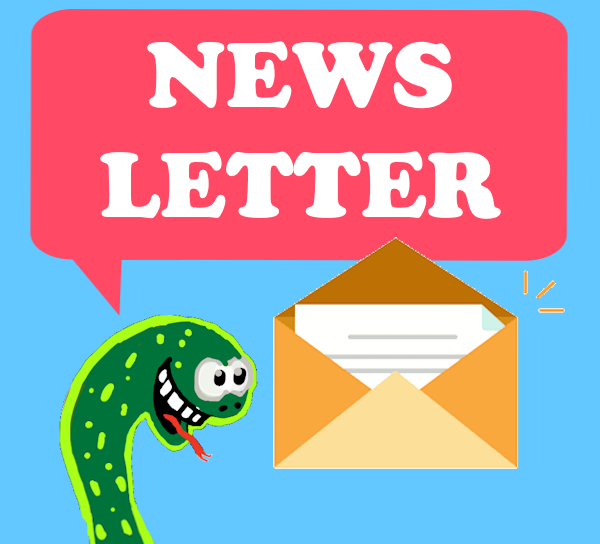


Twitter: @pythonprogrammi - python_pygame
Videos
Speech recognition gamePygame's Platform Game
