Debugging your code is very important, so let’s see 5 tips to do it:
- Use a Debugger: Python has a built-in debugger called pdb that allows you to step through code, inspect variables, and set breakpoints.
- Use print statements: Print statements are a simple but effective way to debug your code. You can print out the values of variables at different points in your code to see how they change as the program executes.
- Check for syntax errors: Syntax errors are the most basic type of error and are usually easy to fix. Make sure to check your code for any syntax errors, as they can be the source of more elusive bugs.
- Test your code in small pieces: Instead of trying to run your entire program at once, try running small pieces of code and testing them individually. This will help you isolate any issues and make it easier to fix them.
- Don’t be afraid to ask for help: If you’re stuck and can’t figure out what’s wrong with your code, don’t be afraid to ask for help. There are many online communities, forums, and groups where you can get help from other Python developers.
I hope these tips are helpful! Let me know if you have any questions.
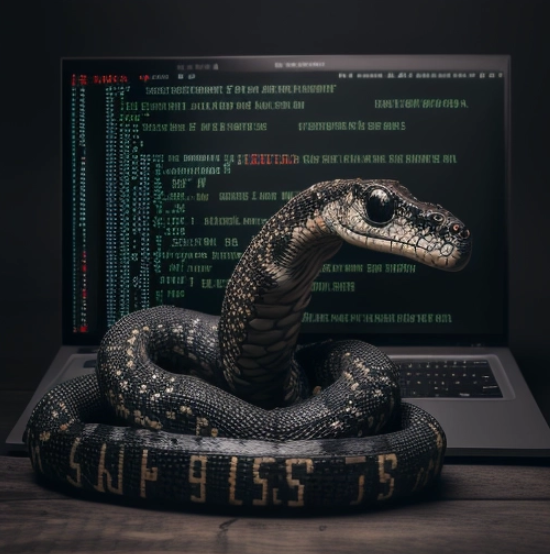
let’s make an example of usage of pdb to debug pytyhon’s code
Here is an example of using the Python debugger (pdb) to debug a simple program:
# Import the pdb module import pdb def add(num1, num2): # Set a breakpoint pdb.set_trace() result = num1 + num2 return result # Call the add function with some arguments result = add(5, 10) print(result)
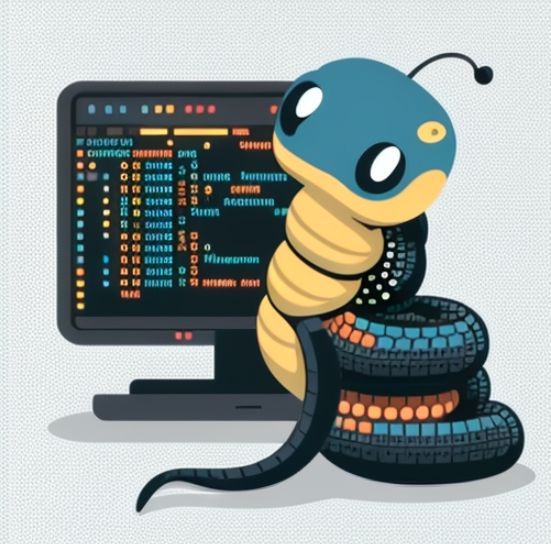
To use the debugger, run the program with the python -m pdb <filename>
command. This will launch the debugger and pause the program at the pdb.set_trace()
line.
Once the program is paused, you can use the following commands to debug:
n
: execute the current line and move to the next lines
: execute the current line and move to the next line, stepping into any function callsc
: continue execution until the next breakpoint or the end of the programq
: quit the debugger and exit the programp <expression>
: print the value of an expressionl
: list the source code for the current fileu <number>
: move up the call stack by the specified number of levels
Here is how you can test the code of the example:
Microsoft Windows [Version 10.0.22000.1335] (c) Microsoft Corporation. All rights reserved. C:\Program Files\Sublime Text\debugger>python -m pdb e01.py > c:\program files\sublime text\debugger\e01.py(2)<module>() -> import pdb (Pdb) n > c:\program files\sublime text\debugger\e01.py(4)<module>() -> def add(num1, num2): (Pdb) n > c:\program files\sublime text\debugger\e01.py(11)<module>() -> result = add(5, 10) (Pdb) n > c:\program files\sublime text\debugger\e01.py(7)add() -> result = num1 + num2 (Pdb) n > c:\program files\sublime text\debugger\e01.py(8)add() -> return result (Pdb) n --Return-- > c:\program files\sublime text\debugger\e01.py(8)add()->15 -> return result (Pdb) n > c:\program files\sublime text\debugger\e01.py(12)<module>() -> print(result) (Pdb) n 15
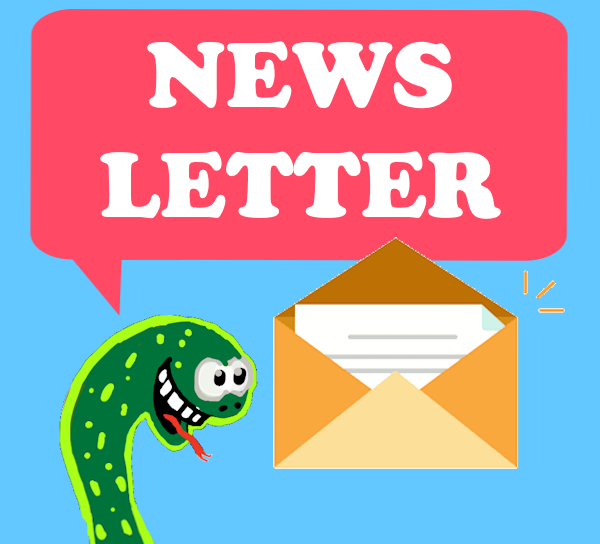


Twitter: @pythonprogrammi - python_pygame
Videos
Speech recognition gamePygame's Platform Game
