Update
The code has been updated, so that it has no problem with the change of the months, as I was using a simple -1 to go back in the days… but it worked only if we were from day 5 on of the month. So I used timedelta(n) to go back of n days (-1…). Here is the code.
import pandas as pd from datetime import datetime, timedelta import matplotlib.pyplot as plt days = [] days2 = [] for n in range(5, 0, -1): day = datetime.today() - timedelta(n) days2.append(str(day.day)) days.append(str(day.month) + "/" + str(day.day)) month = str(datetime.today().month) c = [] def check(what, day): url = "https://raw.githubusercontent.com/CSSEGISandData/COVID-19/master/csse_covid_19_data/csse_covid_19_time_series/time_series_19-covid-{}.csv".format(what) df = pd.read_csv(url, error_bad_lines=False) print(what, end=" ") result = df.loc[df["Country/Region"]=="Italy"]["{}/20".format(day)] print(list(result)[0], end=" - ") if what == "Deaths": c.append(list(result)[0]) what = "Confirmed", "Recovered", "Deaths" for d in days: print("{}".format(d), end=": ") for w in what: check(w, d) print() p = plt.bar(days2, c) plt.xlabel("days: ") plt.ylabel("Deaths") plt.show()
The code to insert in your web page
If you want to make the code working on your web page, use Script n Style plugin (for wordpress) and then paste this into a hoop. If you do not use wordpress, you just put this into the web page.
<script async src="https://cdn.datacamp.com/dcl-react.js.gz"></script> <div class="exercise"> <div class="title"> <h2>Deaths</h2> </div> <div data-datacamp-exercise data-lang="python"> <code data-type="pre-exercise-code"></code> <code data-type="sample-code"> import pandas as pd from datetime import datetime, timedelta import matplotlib.pyplot as plt days = [] days2 = [] for n in range(5, 0, -1): day = datetime.today() - timedelta(n) days2.append(str(day.day)) days.append(str(day.month) + "/" + str(day.day)) month = str(datetime.today().month) c = [] def check(what, day): url = "https://raw.githubusercontent.com/CSSEGISandData/COVID-19/master/csse_covid_19_data/csse_covid_19_time_series/time_series_19-covid-{}.csv".format(what) df = pd.read_csv(url, error_bad_lines=False) print(what, end=" ") result = df.loc[df["Country/Region"]=="Italy"]["{}/20".format(day)] print(list(result)[0], end=" - ") if what == "Deaths": c.append(list(result)[0]) what = "Confirmed", "Recovered", "Deaths" for d in days: print("{}".format(d), end=": ") for w in what: check(w, d) print() p = plt.bar(days2, c) plt.xlabel("days: ") plt.ylabel("Deaths") plt.show() </code> <code data-type="solution"></code> <code data-type="sct"></code> <div data-type="hint">Just press 'Run'.</div> </div> </div>
And now let’s see the code in action.
Check the data live in the shell
English:
Throught the shells you can check the data from this source that are costantly updated about coronavirus. Hit run to see the data. You will get the graph of the confirmed cases, the recovered and the deaths of the last days in Italy. I will make a new post to check different countries too.
Italiano:
Attraverso le sheel puoi verificare i dati (da questa fonte) costantemente aggiornati sul coronavirus. Clicca su run per vedere i dati (attendere qualche secondo per vederli. Vedrai un grafico dei casi confermati, i guariti e i deceduti negli ultimi giorni in Italia. Farò delle modifiche per poter verificare agli gli altri Stati.
Confirmed (confermati/positivi)
Click on run below (clicca sul tasto run per le statistiche)
import pandas as pd
from datetime import datetime, timedelta
import matplotlib.pyplot as plt
days = []
days2 = []
for n in range(5, 0, -1):
day = datetime.today() - timedelta(n)
days2.append(str(day.day))
days.append(str(day.month) + "/" + str(day.day))
month = str(datetime.today().month)
c = []
def check(what, day):
url = "https://raw.githubusercontent.com/CSSEGISandData/COVID-19/master/csse_covid_19_data/csse_covid_19_time_series/time_series_19-covid-{}.csv".format(what)
df = pd.read_csv(url, error_bad_lines=False)
print(what, end=" ")
result = df.loc[df["Country/Region"]=="Italy"]["{}/20".format(day)]
print(list(result)[0], end=" - ")
if what == "Confirmed":
c.append(list(result)[0])
what = "Confirmed", "Recovered", "Deaths"
for d in days:
print("{}".format(d), end=": ")
for w in what:
check(w, d)
print()
p = plt.bar(days2, c)
plt.xlabel("days: ")
plt.ylabel("Confirmed")
plt.show()
Recovered (guariti)
Click on run below (clicca sul tasto run per le statistiche)
import pandas as pd
from datetime import datetime, timedelta
import matplotlib.pyplot as plt
days = []
days2 = []
for n in range(5, 0, -1):
day = datetime.today() - timedelta(n)
days2.append(str(day.day))
days.append(str(day.month) + "/" + str(day.day))
month = str(datetime.today().month)
c = []
def check(what, day):
url = "https://raw.githubusercontent.com/CSSEGISandData/COVID-19/master/csse_covid_19_data/csse_covid_19_time_series/time_series_19-covid-{}.csv".format(what)
df = pd.read_csv(url, error_bad_lines=False)
print(what, end=" ")
result = df.loc[df["Country/Region"]=="Italy"]["{}/20".format(day)]
print(list(result)[0], end=" - ")
if what == "Recovered":
c.append(list(result)[0])
what = "Confirmed", "Recovered", "Deaths"
for d in days:
print("{}".format(d), end=": ")
for w in what:
check(w, d)
print()
p = plt.bar(days2, c)
plt.xlabel("days: ")
plt.ylabel("Recovered")
plt.show()
Deaths (decessi)
Click on run below (clicca sul tasto run per le statistiche)
import pandas as pd
from datetime import datetime, timedelta
import matplotlib.pyplot as plt
days = []
days2 = []
for n in range(5, 0, -1):
day = datetime.today() - timedelta(n)
days2.append(str(day.day))
days.append(str(day.month) + "/" + str(day.day))
month = str(datetime.today().month)
c = []
def check(what, day):
url = "https://raw.githubusercontent.com/CSSEGISandData/COVID-19/master/csse_covid_19_data/csse_covid_19_time_series/time_series_19-covid-{}.csv".format(what)
df = pd.read_csv(url, error_bad_lines=False)
print(what, end=" ")
result = df.loc[df["Country/Region"]=="Italy"]["{}/20".format(day)]
print(list(result)[0], end=" - ")
if what == "Deaths":
c.append(list(result)[0])
what = "Confirmed", "Recovered", "Deaths"
for d in days:
print("{}".format(d), end=": ")
for w in what:
check(w, d)
print()
p = plt.bar(days2, c)
plt.xlabel("days: ")
plt.ylabel("Deaths")
plt.show()
If you want to check another country (like France, for example), change the code here
result = df.loc[df[“Country/Region”]==”Italy”][“{}/{}/20”.format(month, day)]
and put France instead of Italy. You can also check South Korea. For China or USA is a little more complicated, because there are more rows for these countries.
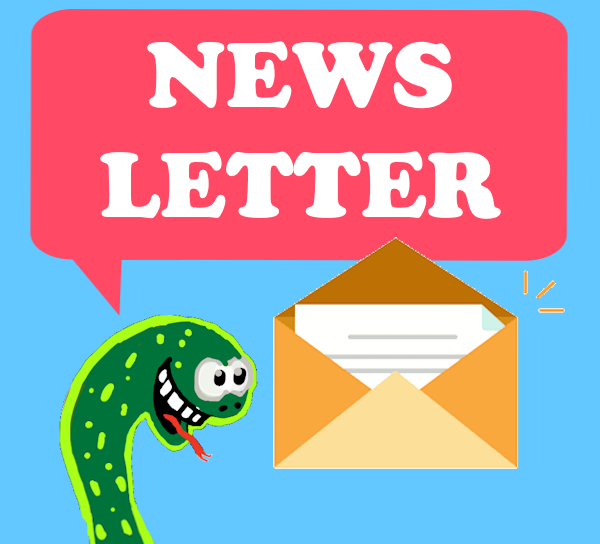


Twitter: @pythonprogrammi - python_pygame
Videos
Speech recognition gamePygame's Platform Game
