To convert an image in color into an image in black an white you can use this code, that uses just the PIL module.
from PIL import Image col = Image.open("man.png") gray = col.convert('L') bw = gray.point(lambda x: 0 if x<128 else 255, '1') bw.save("man_bn.png")
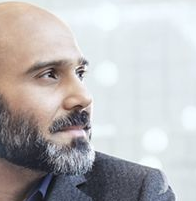
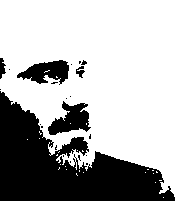
Youn can also get different type of convertions with this code
from PIL import Image import glob import os cnt = 1 pics = glob.glob("*.png") for n in range(10,255,25): for pic in pics: col = Image.open(pic) gray = col.convert('L') bw = gray.point(lambda x: 0 if x<n else 255, '1') if cnt < 100: pre = 0 else: pre = "" bw = bw.convert("RGB", palette=Image.ADAPTIVE, colors=8) bw.save(f"{pic[:-4]}{pre}{cnt}.png") os.startfile(f"{pic[:-4]}{pre}{cnt}.png") cnt += 1
I have re-converted to RGB the files, after the convertion in black and white (you will see the reason below).
This is the result
You could also use this code to create an animation with the changing ‘shadows’ (and this is the reason for I re-converted to RGB the images, before I saved them, otherwise I would have had an error in the following code due to the wrong color palette.
from PIL import Image import glob import os # Create the frames frames = [] imgs = glob.glob("*.png") for i in imgs: new_frame = Image.open(i) frames.append(new_frame) # Save into a GIF file that loops forever frames[0].save('png_to_gif.gif', format='GIF', append_images=frames[1:], save_all=True, duration=300, loop=0) os.startfile("png_to_gif.gif")
This is the output
That is all for now… to be continued?