Countries with more confirmed cases outside China
People tasted each day / I tamponi effettuati
Follow the evolution of the coronavirus from the official data. You get the data about the last 5 days each time you hit run in the shell below the code. You will always get the last 5 day, not included today.
Today I am gonna show only the data in the online shell. But, do not worry, here is the code:
<script async src="https://cdn.datacamp.com/dcl-react.js.gz"></script> <div class="exercise"> <div class="title"> <h2>Deaths</h2> </div> <div data-datacamp-exercise data-lang="python"> <code data-type="pre-exercise-code"> import pandas as pd from datetime import datetime, timedelta import matplotlib.pyplot as plt days = [] days2 = [] for n in range(5, 0, -1): day = datetime.today() - timedelta(n) days2.append(str(day.day)) days.append(str(day.month) + "/" + str(day.day)) month = str(datetime.today().month) c = [] def check(what, day): url = "https://raw.githubusercontent.com/CSSEGISandData/COVID-19/master/csse_covid_19_data/csse_covid_19_time_series/time_series_19-covid-{}.csv".format(what) df = pd.read_csv(url, error_bad_lines=False) print(what, end=" ") result = df.loc[df["Country/Region"]=="Italy"]["{}/20".format(day)] print(list(result)[0], end=" - ") if what == "Deaths": c.append(list(result)[0]) what = "Confirmed", "Recovered", "Deaths" for d in days: print("{}".format(d), end=": ") for w in what: check(w, d) print() p = plt.bar(days2, c) plt.xlabel("days: ") plt.ylabel("Deaths") plt.show() </code> <code data-type="sample-code"> </code> <code data-type="solution"></code> <code data-type="sct"></code> <div data-type="hint">Just press 'Run'.</div> </div> </div>
We can check some data here. Press RUN to see the graph of the data.
Premere RUN per vedere i dati. Attendi qualche istante.
Confirmed in Italy / Casi confermati in Italia
import pandas as pd
from datetime import datetime, timedelta
import matplotlib.pyplot as plt
days = []
days2 = []
for n in range(5, 0, -1):
day = datetime.today() - timedelta(n)
days2.append(datetime(2020, day.month, day.day))
days.append(str(day.month) + "/" + str(day.day))
month = str(datetime.today().month)
c = []
def check(what, day):
url = "https://raw.githubusercontent.com/CSSEGISandData/COVID-19/master/csse_covid_19_data/csse_covid_19_time_series/time_series_19-covid-{}.csv".format(what)
df = pd.read_csv(url, error_bad_lines=False)
print(what, end=" ")
result = df.loc[df["Country/Region"]=="Italy"]["{}/20".format(day)]
print(list(result)[0], end=" - ")
if what == "Confirmed":
c.append(list(result)[0])
what = "Confirmed", "Recovered", "Deaths"
for d in days:
print("{}".format(d), end=": ")
for w in what:
check(w, d)
print()
#sorted(days, key=lambda d: map(int, d.split('/')))
ax = plt.subplot(111)
ax.bar(days2, c)
ax.xaxis_date()
plt.xlabel("days: ")
plt.ylabel("Confirmed / positivi")
plt.show()
# press RUN below to see the data
Recovered in Italy / Guariti in Italia
import pandas as pd
from datetime import datetime, timedelta
import matplotlib.pyplot as plt
days = []
days2 = []
for n in range(5, 0, -1):
day = datetime.today() - timedelta(n)
days2.append(datetime(2020, day.month, day.day))
days.append(str(day.month) + "/" + str(day.day))
month = str(datetime.today().month)
c = []
def check(what, day):
url = "https://raw.githubusercontent.com/CSSEGISandData/COVID-19/master/csse_covid_19_data/csse_covid_19_time_series/time_series_19-covid-{}.csv".format(what)
df = pd.read_csv(url, error_bad_lines=False)
print(what, end=" ")
result = df.loc[df["Country/Region"]=="Italy"]["{}/20".format(day)]
print(list(result)[0], end=" - ")
if what == "Recovered":
c.append(list(result)[0])
what = "Confirmed", "Recovered", "Deaths"
for d in days:
print("{}".format(d), end=": ")
for w in what:
check(w, d)
print()
#sorted(days, key=lambda d: map(int, d.split('/')))
ax = plt.subplot(111)
ax.bar(days2, c)
ax.xaxis_date()
plt.xlabel("days: ")
plt.ylabel("Recovered / guariti")
plt.show()
# press RUN below to see the data
Confirmed in France
import pandas as pd
from datetime import datetime, timedelta
import matplotlib.pyplot as plt
days = []
days2 = []
for n in range(5, 0, -1):
day = datetime.today() - timedelta(n)
days2.append(datetime(2020, day.month, day.day))
days.append(str(day.month) + "/" + str(day.day))
month = str(datetime.today().month)
c = []
def check(what, day):
url = "https://raw.githubusercontent.com/CSSEGISandData/COVID-19/master/csse_covid_19_data/csse_covid_19_time_series/time_series_19-covid-{}.csv".format(what)
df = pd.read_csv(url, error_bad_lines=False)
print(what, end=" ")
result = df.loc[df["Country/Region"]=="France"]["{}/20".format(day)]
print(list(result)[0], end=" - ")
if what == "Confirmed":
c.append(list(result)[0])
what = "Confirmed", "Recovered", "Deaths"
for d in days:
print("{}".format(d), end=": ")
for w in what:
check(w, d)
print()
#sorted(days, key=lambda d: map(int, d.split('/')))
ax = plt.subplot(111)
ax.bar(days2, c)
ax.xaxis_date()
plt.xlabel("days: ")
plt.ylabel("Confirmed / positivi")
plt.show()
# press RUN below to see the data
Confirmed in South Korea
import pandas as pd
from datetime import datetime, timedelta
import matplotlib.pyplot as plt
days = []
days2 = []
for n in range(5, 0, -1):
day = datetime.today() - timedelta(n)
days2.append(datetime(2020, day.month, day.day))
days.append(str(day.month) + "/" + str(day.day))
month = str(datetime.today().month)
c = []
def check(what, day):
url = "https://raw.githubusercontent.com/CSSEGISandData/COVID-19/master/csse_covid_19_data/csse_covid_19_time_series/time_series_19-covid-{}.csv".format(what)
df = pd.read_csv(url, error_bad_lines=False)
print(what, end=" ")
result = df.loc[df["Country/Region"]=="South Korea"]["{}/20".format(day)]
print(list(result)[0], end=" - ")
if what == "Confirmed":
c.append(list(result)[0])
what = "Confirmed", "Recovered", "Deaths"
for d in days:
print("{}".format(d), end=": ")
for w in what:
check(w, d)
print()
#sorted(days, key=lambda d: map(int, d.split('/')))
ax = plt.subplot(111)
ax.bar(days2, c)
ax.xaxis_date()
plt.xlabel("days: ")
plt.ylabel("Confirmed / positivi")
plt.show()
# press RUN below to see the data
Confirmed Iran
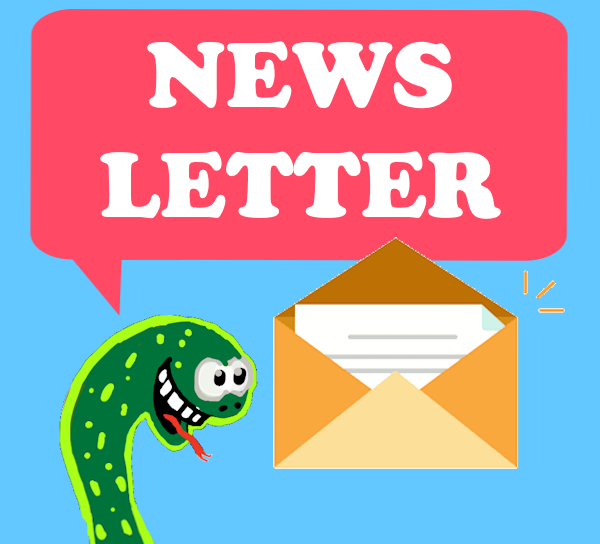


Twitter: @pythonprogrammi - python_pygame
Videos
Speech recognition gamePygame's Platform Game
