The whole code
With this code we can create our table in html, starting from a table made in Excel like this:
then, due to the need to convert this table in an html table, we copy it (ctrl+c) and paste it into our application. Then we press convert, then we save it and we show the table with the menu command save and show.
Here is the GUI when we copied the Excel data:
Then we press convert and we have this:
You can see the data converted into html table. You then save and show the html file with the two commands in the menu. This is the output (the one above is not a picture, we used the html code generated above)
N. | Bene_A_4% | Bene_B_10% | Trasporto | CDR | Trasp_A | trasp_B | IVA_A | IVA_B | TOT |
1 | 1200,00 | 800,00 | 100,00 | 0,05 | 60,00 | 40,00 | 50,40 | 84,00 | 2234,40 |
2 | 1400,00 | 900,00 | 120,00 | 0,05 | 73,04 | 46,96 | 58,92 | 94,70 | 2573,62 |
3 | 1600,00 | 1000,00 | 140,00 | 0,05 | 86,15 | 53,85 | 67,45 | 105,38 | 2912,83 |
4 | 1800,00 | 1100,00 | 160,00 | 0,06 | 99,31 | 60,69 | 75,97 | 116,07 | 3252,04 |
5 | 2000,00 | 1200,00 | 180,00 | 0,06 | 112,50 | 67,50 | 84,50 | 126,75 | 3591,25 |
The whole code
This is the whole code to make this simple but useful app.
import os html = "" def table(x): global html """ Create table with data in a multiline string as first argument x)""" html = "<!-- table -->" html += "<table border=1>" for line in x.splitlines(): for n in line.split(): html += f"<td>{n}</td>" html += "<tr>" html += "</table>" return html def create(a): tab = table(text.get("1.0", tk.END)) text.delete("1.0", tk.END) text.insert("1.0", tab) label['text'] = "Now you can copy the html code for the table (ctrl + a)" def save_html(): if html != "": with open("table.html", "w") as file: file.write(text.get("1.0", tk.END)) def show_html(): if os.path.exists("table.html"): os.startfile("table.html") def convert_to_html(): html = table(text.get("1.0",tk.END)) clear() text.insert("1.0", html) def clear(): text.delete("1.0", tk.END) import tkinter as tk root = tk.Tk() root.title("Html table converter") label = tk.Label(root, text="Insert data here separated by space and press Ctrl+c to convert to html table:") label.pack() text = tk.Text(root) text.pack() text.bind("<Control-c>", create) text.focus() # create a toplevel menu menubar = tk.Menu(root) menubar.add_command(label="Convert - crtl+c |", command=convert_to_html) menubar.add_command(label="Save |", command=save_html) menubar.add_command(label="Show |", command=show_html) menubar.add_command(label="Clear screen |", command=clear) # display the menu root.config(menu=menubar) root.mainloop()
*update: addet crtl+c as shortcut to convert data into html
Version 2
In this version we have two text box, the first for the text, the second with the html. In this version you separare the cells with tabs, so that you can use a space between words in the same cell (otherwise, it would separate each word into different cells, having you to write two words like this first_second, to avoid splitting the single words into different cells.
The code:
import os html = "" def table(x): global html html = "" """ Create table with data in a multiline string as first argument x)""" html = "<!-- table -->" html += "<table border=1>" for line in x.splitlines(): for n in line.split("\t"): html += f"<td>{n}</td>" html += "<tr>" html += "</table>" return html def create(a): tab = table(text.get("1.0", tk.END)) #text.delete("1.0", tk.END) text2.insert("1.0", tab) label['text'] = "Now you can copy the html code for the table (ctrl + a)" def save_html(): if html != "": with open("table.html", "w", encoding="utf-8") as file: file.write(text2.get("1.0", tk.END)) def show_html(): if os.path.exists("table.html"): os.startfile("table.html") def convert_to_html(): html = table(text.get("1.0",tk.END)) clear2() text2.insert("1.0", html) def clear2(): text2.delete("1.0", tk.END) def clear(): text.delete("1.0", tk.END) text2.delete("1.0", tk.END) import tkinter as tk root = tk.Tk() root.title("Html table converter") label = tk.Label(root, text="Insert data here separated by tabs") label.pack() text = tk.Text(root, height=7) text.pack() text.bind("<Control-p>", create) text.focus() label2 = tk.Label(root, text="Press ctr+p and you'll get the html code here") label2.pack() text2 = tk.Text(root, bg='gold', height=7) text2.pack() # create a toplevel menu menubar = tk.Menu(root) menubar.add_command(label="Convert - crtl+p |", command=convert_to_html) menubar.add_command(label="Save |", command=save_html) menubar.add_command(label="Show |", command=show_html) menubar.add_command(label="Clear screen |", command=clear) # display the menu root.config(menu=menubar) root.mainloop()
Version 3: filedialog
Now you can open a txt file with the data from a filedialog window called by tkinter. This way, you can save your data into a txt file, so that you can change them and convert them easily with the app into a html table. Now it is also possible to clean the html tags and get back the cleaned data, pasting (or opening) a html code/file.
import tkinter as tk import os from tkinter import filedialog my_filetypes = [('python', '.py'), ('html', '.html'), ('text files', '.txt')] def open_folder(): answer = filedialog.askopenfilename(parent=root, initialdir=os.getcwd(), title="Please select a file:", filetypes=my_filetypes) try: text.delete("1.0", tk.END) with open(answer) as file: text.insert("1.0", file.read()) except FileNotFoundError: print("file not found") def convert_reverse(x): tags = [ ("</table>",""), ("<!-- table -->",""), ("<table border=1>",""), ("<td>","\t"), ("</td>", ""), ("<tr>", "\n")] for tag in tags: x = x.replace(tag[0], tag[1]) return x html = "" def table(x): global html if "<table" in x: return convert_reverse(x) html = "" """ Create table with data in a multiline string as first argument x)""" html += "<table border=1>" for line in x.splitlines(): for n in line.split("\t"): if n == "": continue html += f"<td>{n}</td>" html += "<tr>" html += "</table>" return html def create(a): tab = table(text.get("1.0", tk.END)) #text.delete("1.0", tk.END) text2.insert("1.0", tab) label['text'] = "Now you can copy the html code for the table (ctrl + a)" def save_html(): if html != "": with open("table.html", "w", encoding="utf-8") as file: file.write(text2.get("1.0", tk.END)) def show_html(): if os.path.exists("table.html"): os.startfile("table.html") def convert_to_html(): html = table(text.get("1.0",tk.END)) clear2() text2.insert("1.0", html) def clear2(): text2.delete("1.0", tk.END) def clear(): text.delete("1.0", tk.END) text2.delete("1.0", tk.END) root = tk.Tk() root.title("Html table converter") label = tk.Label(root, text="Copy data from Excel and insert them here separated by tabs") label.pack() text = tk.Text(root) text.pack(fill=tk.BOTH, expand=1) text.bind("<Control-p>", create) text.focus() label2 = tk.Label(root, text="Press ctr+p and you'll get the html code here") label2.pack() text2 = tk.Text(root, bg='gold') text2.pack(fill=tk.BOTH, expand=1) # create a toplevel menu menubar = tk.Menu(root) menubar.add_command(label="Open", command=open_folder) menubar.add_command(label="Convert", command=convert_to_html) menubar.add_command(label="Save", command=save_html) menubar.add_command(label="Show", command=show_html) menubar.add_command(label="Clear", command=clear) # display the menu root.config(menu=menubar) root.mainloop()
Tkinter test for students
Tkinter articles
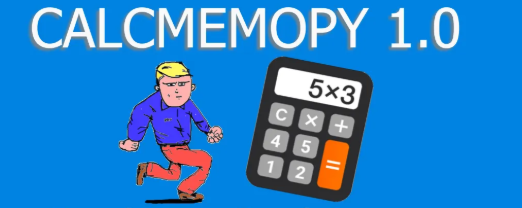