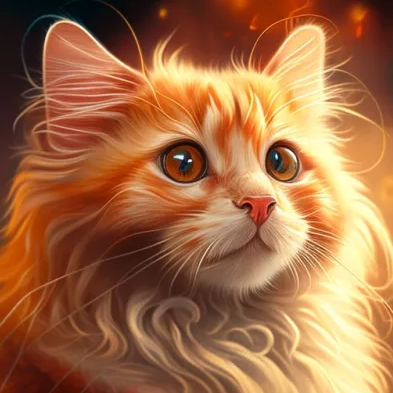
create a script in python to recognize if in a photo there is a cat or a dog
Here is a simple script in Python that uses the OpenCV library to detect whether there is a cat or a dog in a photo:
import cv2 # Load the cascade classifiers for cats and dogs cat_cascade = cv2.CascadeClassifier('cat.xml') # dog_cascade = cv2.CascadeClassifier('dog.xml') # Read the image img = cv2.imread('cat.jpg') # Convert the image to grayscale gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY) # Detect cats and dogs in the image cats = cat_cascade.detectMultiScale(gray, 1.1, 3) # dogs = dog_cascade.detectMultiScale(gray, 1.1, 3) # Print the number of cats and dogs detected print("Cats detected: ", len(cats)) # print("Dogs detected: ", len(dogs))
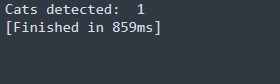
This script reads an image from a file, converts it to grayscale, and then uses the detectMultiScale function of the CascadeClassifier object to detect the presence of cats or dogs in the image. The detectMultiScale function returns a list of rectangles, which are the bounding boxes around the detected objects. The script then prints the number of cats and dogs detected in the image. You will need to have the OpenCV library installed, as well as the cat.xml and dog.xml cascade classifiers. You can download the classifiers from the OpenCV repository or use your own trained classifiers.
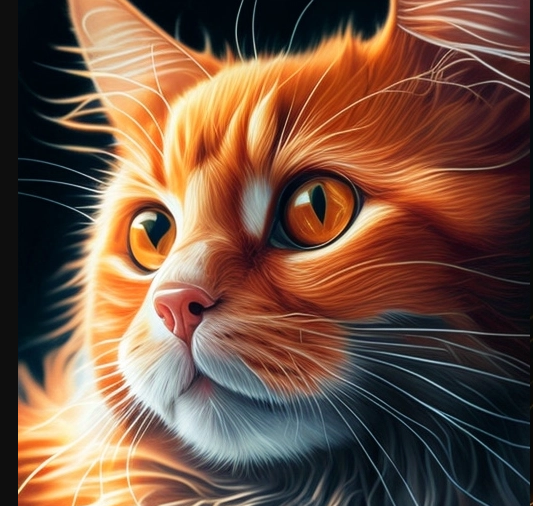
The data The cat.xml and dog.xml files are cascade classifiers that are used by the OpenCV library to detect objects in images. These classifiers are trained using machine learning algorithms to recognize certain patterns in images. You can find the cat.xml and dog.xml files in the data folder of the OpenCV repository on GitHub. Here is the link to the repository: https://github.com/opencv/opencv/tree/master/data You can download the classifiers from the repository and then use them in your Python script by specifying the path to the classifier file. Alternatively, you can also train your own cascade classifiers using the OpenCV library. To do this, you will need a large dataset of images of cats and dogs, and a set of positive and negative samples for training. You can then use the opencv_traincascade command-line tool to train the classifier. Another type of code could be this
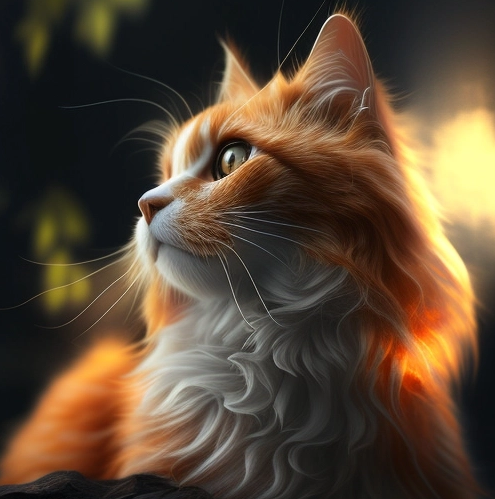
import cv2 # read the input image img = cv2.imread('cat.jpg') # convert the input image to grayscale gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY) # read the haarcascade to detect cat faces cat_cascade = cv2.CascadeClassifier('cat.xml') # Detects cat faces in the input image faces = cat_cascade.detectMultiScale(gray, 1.1, 3) print('Number of detected cat faces:', len(faces)) # if atleast one cat face id detected if len(faces) > 0: print("Cat face detected") for (x,y,w,h) in faces: # To draw a rectangle in a face cv2.rectangle(img,(x,y),(x+w,y+h),(0,255,255),2) cv2.putText(img, 'cat face', (x, y-3), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0,255,0), 1) else: print("No cat face detected") # Display an image in a window cv2.imshow('Cat Image',img) cv2.waitKey(0) cv2.destroyAllWindows()
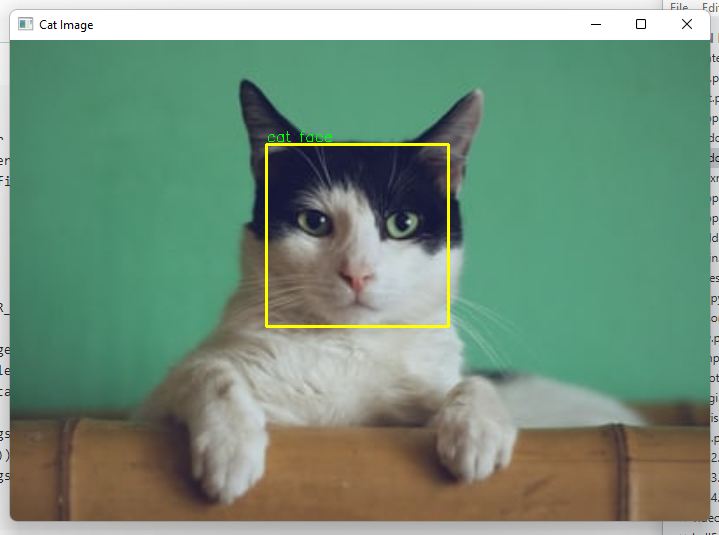
The cat.xml (I renamed it) can be fount here https://raw.githubusercontent.com/opencv/opencv/master/data/haarcascades/haarcascade_frontalcatface.xml
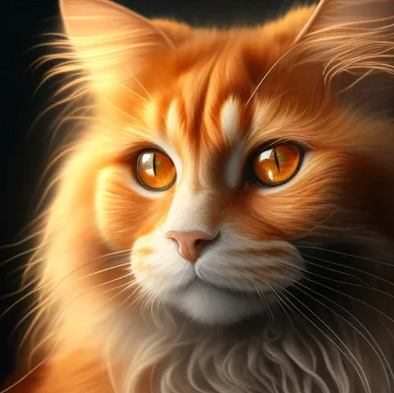
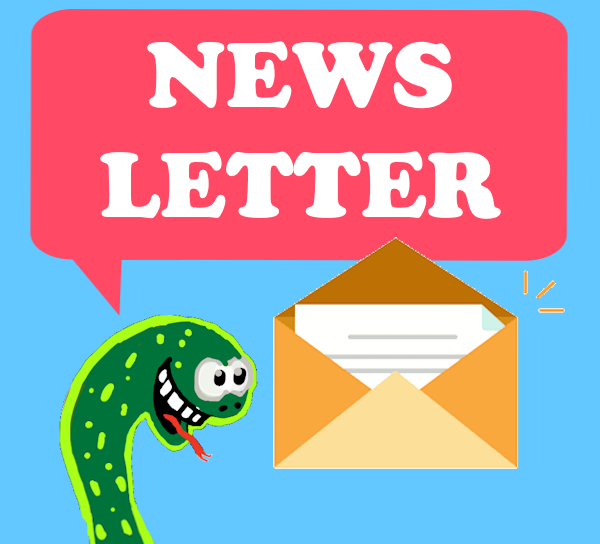


Twitter: @pythonprogrammi - python_pygame
Videos
Speech recognition gamePygame's Platform Game
