First create a window with tkinter
import tkinter as tk import time root = tk.Tk() root.title("Window and menubar") root.geometry("400x400+100+100") root.mainloop()
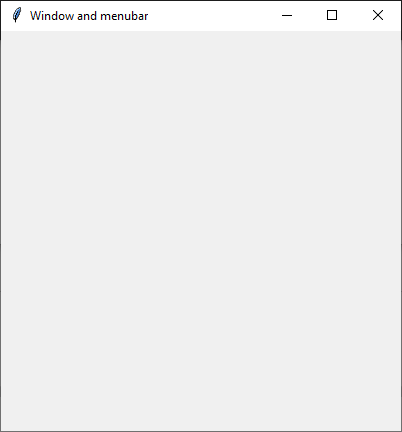
Add a menu to the window
import tkinter as tk import time root = tk.Tk() root.title("Window and menubar") root.geometry("400x400+100+100") menubar = tk.Menu(root) menubar.add_command(label="Click me", command=None) root.config(menu=menubar) root.mainloop()
Adding a command to the menu
import tkinter as tk import time from tkinter import messagebox root = tk.Tk() root.title("Window and menubar") root.geometry("400x400+100+100") def message(): messagebox.showinfo("MESSAGE", "This is pythonprogramming.altervista.org", parent=root) menubar = tk.Menu(root) menubar.add_command(label="Click me", command=message) root.config(menu=menubar) root.mainloop()
See ya in another post.
Adding another command to the menu
import tkinter as tk from tkinter import messagebox import os popup = messagebox.showinfo def message(): "Message shown by the menu item 'About' " popup("Hello", "Visit my site") def visit(): "Message shown by the menu item 'About' " os.startfile("https://pythonprogramming.altervista.org") root = tk.Tk() root.title("Window and menubar") root.geometry("400x400+100+100") # create the menu menubar = tk.Menu() root.config(menu=menubar) # adding a voice to the menu with add_command menubar.add_command(label="About", command=message) menubar.add_command(label="Visit", command=visit) root.mainloop()
Video
Twitter: @pythonprogrammi - python_pygame