import pygame class Color: green = (0, 255, 0) class Font: ''' definition of type of fonts ''' pygame.font.init() little = pygame.font.SysFont("Arial", 48) big = pygame.font.SysFont("Arial", 192) class Text: ''' definition of text with rendering method ''' def text_render(font, text, color): return font.render(text, 1, color) def show_text(): screen.blit(Text.hello, (0, 0)) screen.blit(Text.World, (0, 200)) hello = text_render(Font.big, "Hello", Color.green) World = text_render(Font.little, "World", Color.green) def monitor_size(): ''' return width and height of the monitor screen ''' info = pygame.display.Info() return info.current_w, info.current_h def mainloop(): ''' main loop to show stuff on the screen ''' while True: for event in pygame.event.get(): if event.type == pygame.QUIT: pygame.quit() Text.show_text() clock.tick(60) pygame.display.update() pygame.init() w, h = monitor_size() screen = pygame.display.set_mode((w - 100, h - 100), pygame.RESIZABLE) clock = pygame.time.Clock() mainloop()
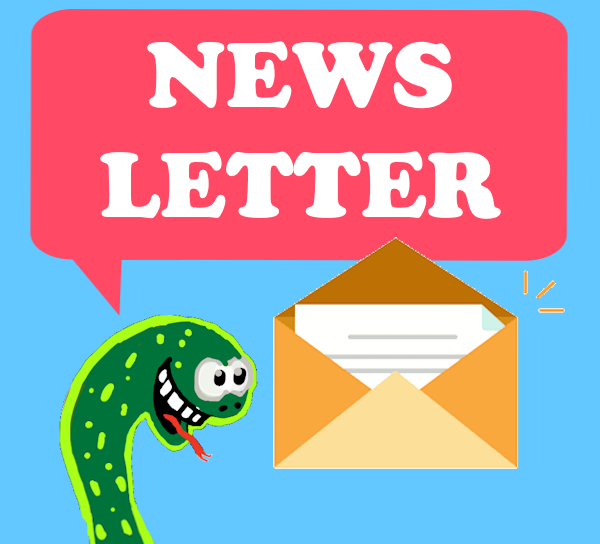


Twitter: @pythonprogrammi - python_pygame
Videos
Speech recognition gamePygame's Platform Game
