In the following video we will get how to create databases with sqlite3 and python. The code that will result will be the following
import sqlite3 as lite conn = lite.connect("db01.db") print(conn) # Create a table tablename = "profile" def create_table(): cur = conn.cursor() command = "CREATE TABLE IF NOT EXISTS" fields = "id INTEGER PRIMARY KEY, name TEXT, surname TEXT" cur.execute(f"{command} {tablename}({fields})") conn.commit() def insert_data(): data = ( ("John", "Smith"), ("Jane", "Austin") ) cur = conn.cursor() command = "INSERT INTO" fields = "id, name, surname" for n, d in enumerate(data): name, surname = d cur.execute(f"{command} {tablename}({fields}) VALUES ({n}, '{name}', '{surname}')") conn.commit() def show_data(): cur = conn.cursor() cur.execute("SELECT * FROM " + tablename) rows = cur.fetchall() for r in rows: print(r) show_data() conn.close()
So we can:
- create a database
- create a table
- insert data
- see data

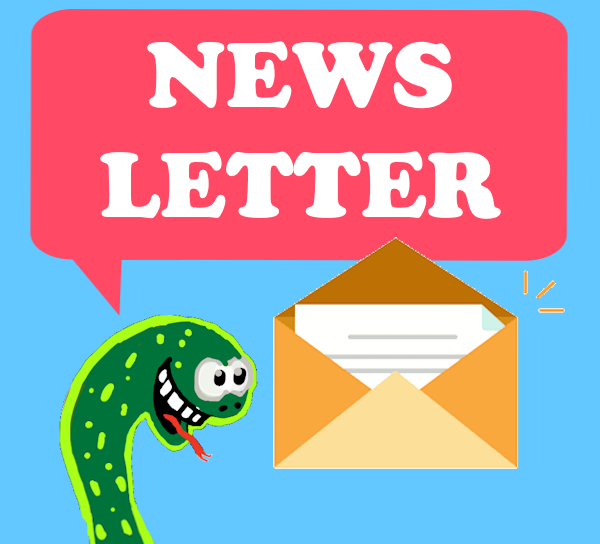


Twitter: @pythonprogrammi - python_pygame
Videos
Speech recognition gamePygame's Platform Game
