

To create a text you need the
- class SysFont to which you pass the “Arial” , 20 (for example) as font and size
- then we create a simple class to render the text with font.render from the SysFont class method
- we create an istance of the Text widget passing the text and the position (eventually the color)
- then we show the text with the update method that blits the text image on the screen (win)
import pygame import random pygame.init() win = pygame.display.set_mode((600, 600)) clock = pygame.time.Clock() font = pygame.font.SysFont("Arial", 20) class Text: ''' CLASS FOR TEXT LABELS ON THE WIN SCREEN SURFACE ''' def __init__(self, text, x, y, color="white"): self.image = font.render(text, 1, color) _, _, w, h = self.image.get_rect() self.rect = pygame.Rect(x, y, w, h) def draw(self): win.blit(self.image, (self.rect)) # TEXT TO SHOW ON THE SCREEN AT POS 100 100 score = Text("Ciao a tutti", 100, 100) # LOOP TO MAKE THINGS ON THE SCRREEN loop = 1 while loop: win.fill(0) # CLEAN THE SCREEN EVERY FRAME # CODE TO CLOSE THE WINDOW for event in pygame.event.get(): if event.type == pygame.QUIT: loop = 0 if event.type == pygame.KEYDOWN: if event.key == pygame.K_ESCAPE: loop = 0 # CODE TO SHOW TEXT EVERY FRAME score.draw() pygame.display.update() clock.tick(60) pygame.quit()
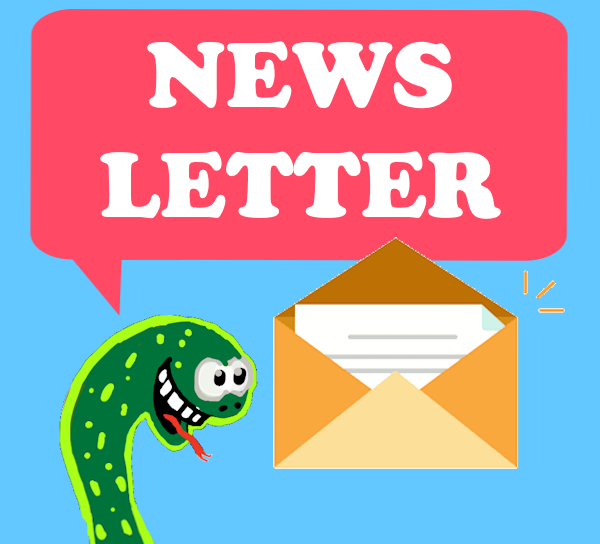


Twitter: @pythonprogrammi - python_pygame
Videos
Speech recognition gamePygame's Platform Game
