Let’s see how can we solve a problem automatically thanks to python getting the data from a text and making also the computer to find the solution for you, using the modile re for regex (tecnique to extract data from strings).

We will get a Break even point problem for example. Let’s see how to do it, step by step.
Find all numbers in a text (\d+)

import re tmp = ''' Find q with: - p = 10 € - cv = 5 € CF = 3000 € solution = €€€ ''' data = re.findall(r"\d+", tmp) print(data)
The output

A nice way to use the example above with the f string, notice that with the = symbol you can easily get what the value is related to.
import re tmp = ''' Find q with: - p = 10 € - cv = 5 € CF = 3000 € solution = €€€ ''' data = re.findall(r"\d+", tmp) p, cv, CF = data print(f"{p=}\n{cv=}\n{CF=}")
output

And you can also use the data to find a solution. In this case you got the fixed costs (CF) and the price and cost of the variable costs, so that you can find the break even point, that is the amount of sellings that make an enterprice to recover all the costs.
p, cv, CF = [int(x) for x in data] print(f"{p=}\n{cv=}\n{CF=}") solution = CF / (p -cv) print(solution) print("This is the number of products you have to sell so that the revenues are equal to the costs.")
The output would be this

Let’s make the exercise customizable
import re def ex1(p=10, cv=5, CF=3000, sol=0): ''' solve a bep problem ''' tmp = f'''Find q with: - p = {p} € - cv = {cv} € - CF = {CF} € solution = €€€ ''' pattern = r"(\d+(?:\.\d+)?)" data = re.findall(pattern, tmp) print(data) p, cv, CF = [float(x) for x in data] print(f"{p=}\n{cv=}\n{CF=}") solution = int(CF / (p -cv)) # print the solution if you pass sol=1 if sol: tmp = tmp.replace("€€€", str(solution)) print(tmp) ex1()
If we call the ex1() function without arguments, the results will be the same, but if we give the function some arguments like this:
ex1(p=12, cv=8.5, CF=100000, sol=1)

You can now put more call to the function with different data as argument for different solutions.
Making random exercises for students with solution
This is to make random exercises. Let’s start
import re import random def ex1(p=10, cv=5, CF=3000, sol=0): ''' solve a bep problem ''' tmp = f'''Trova il BEP sapendo che: - p = {p} € - cv = {cv} € - CF = {CF} € solution = €€€ ''' pattern = r"(\d+(?:\.\d+)?)" data = p, cv, CF = re.findall(pattern, tmp) # print(f"{p=} {cv=} {CF=}") p, cv, CF = [float(x) for x in data] # print(f"{p=} €\n{cv=} €\n{CF=} €") solution = int(CF / (p -cv)) # print the solution if you pass sol=1 if sol: tmp = tmp.replace("€€€", str(solution)) print(tmp) def randomize(number): for r in range(number): p = random.randint(10, 20) cv = random.randint(p-10, p-5) CF = random.randrange( (p-cv)*random.randrange(1000, 2000, 100), (p-cv)*random.randrange(4000, 20000, 100), 100 ) ex1(p, cv, CF, sol=1) # ex1() # ex1(p=12, cv=8.5, CF=100000, sol=1) randomize(3)
Output

Transform it in a quiz
import re import random def ex1(p=10, cv=5, CF=3000, sol=0, quiz=0): ''' solve a bep problem ''' tmp = f'''Trova il BEP sapendo che: - p = {p} € - cv = {cv} € - CF = {CF} € [sol: ...........] ''' pattern = r"(\d+(?:\.\d+)?)" data = p, cv, CF = re.findall(pattern, tmp) # print(f"{p=} {cv=} {CF=}") p, cv, CF = [float(x) for x in data] # print(f"{p=} €\n{cv=} €\n{CF=} €") solution = int(CF / (p -cv)) # print the solution if you pass sol=1 if quiz: print(tmp) ask(solution) else: if sol: tmp = tmp.replace("sol: ...........", "Soluzione: " + str(solution)) print(tmp) else: print(tmp) def randomize(number=0, quiz=0, sol=0): for r in range(number): p = random.randint(10, 20) cv = random.randint(p-10, p-5) CF = random.randrange( (p-cv)*random.randrange(1000, 2000, 100), (p-cv)*random.randrange(4000, 20000, 100), 100 ) ex1(p, cv, CF, sol, quiz) def ask(solution): yousol = input("Sol:") if yousol == str(solution): print("Good job!") else: print("No, it's wrong") print(f"{solution=}\n") # ex1() # ex1(p=12, cv=8.5, CF=100000, sol=1) randomize(3, quiz=0, sol=0)
Let’s make it simple
We do not need the re module anymore.
import random def ex1(p=10, cv=5, CF=3000, sol=0, quiz=0): ''' solve a bep problem ''' tmp = f'''Trova il BEP sapendo che: - p = {p} € - cv = {cv} € - CF = {CF} € [sol: ...........] ''' solution = int(CF / (p -cv)) # print the solution if you pass sol=1 if quiz: print(tmp) ask(solution) else: if sol: tmp = tmp.replace("sol: ...........", "Soluzione: " + str(solution)) print(tmp) else: print(tmp) def randomize(number=0, quiz=0, sol=0): for r in range(number): p = random.randint(10, 20) cv = random.randint(p-10, p-5) CF = random.randrange( (p-cv)*random.randrange(1000, 2000, 100), (p-cv)*random.randrange(4000, 20000, 100), 100 ) ex1(p, cv, CF, sol, quiz) def ask(solution): yousol = input("Sol:") if yousol == str(solution): print("Good job!\n") else: print("No, it's wrong") print(f"{solution=}\n") # ex1() # ex1(sol=1) # ex1(p=12, cv=8.5, CF=100000, sol=1) randomize(3, quiz=1, sol=1)
How you can use it
ex1() | the example with default data |
ex1(sol=1) | same as above, but with the solution |
ex1(p=12, cv=10, CF=2000, sol=1) | custom exercise p = price cv = variable costs CF = fixed costs sol=1 # to show the solution |
randomize(3, sol=1) | creates 3 random exercizes with solution |
randomize(3, quiz=1) | Makes a quiz with 3 problems to solve |
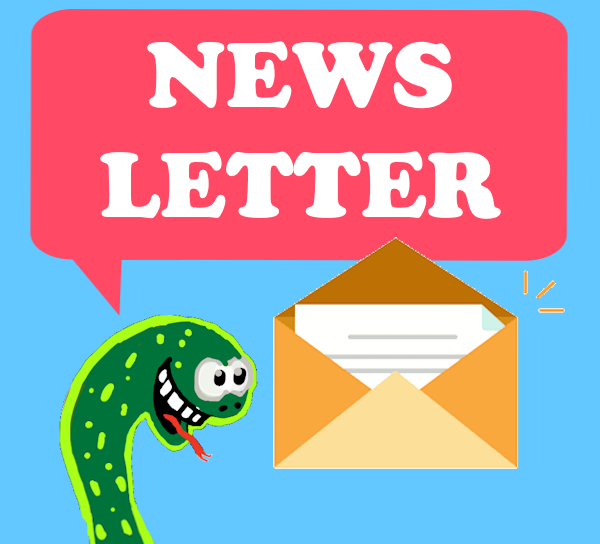


Twitter: @pythonprogrammi - python_pygame
Videos
Speech recognition gamePygame's Platform Game
