A simple app to start videos using tkinter to click on the mp4 to be launched.
import tkinter as tk
import os, glob
root = tk.Tk()
lb = tk.Listbox(root)
lb.pack()
for f in os.listdir():
if f.endswuith(".mp4"):
lb.insert(0, f)
files = glob.glob("*.mp4")
root.mainloop()

Now to start the videos we need to bind the double click to the window.
def launch_mp4(event):
cur = lb.curselection()
get_filename = lb.get(cur)
os.startfile(get_filename)
lb.bind("", launch_mp4)
This will do the work.
To launch a video from youtube

def launch_youtube(event):
url = entry.get()
os.system(f"start {url}")
lab = tk.Label(root, text="Enter youtube url").pack()
entry = tk.Entry(root)
entry.pack()
entry.bind("", launch_youtube)
The whole code
import tkinter as tk
import os, glob
root = tk.Tk()
lb = tk.Listbox(root)
lb.pack()
[lb.insert(0, f) for f in os.listdir() if f.endswith(".mp4")]
files = glob.glob("*.mp4")
def launch_mp4(event):
cur = lb.curselection()
get_filename = lb.get(cur)
os.startfile(get_filename)
lb.bind("", launch_mp4)
def launch_youtube(event):
url = entry.get()
os.system(f"start {url}")
lab = tk.Label(root, text="Enter youtube url").pack()
entry = tk.Entry(root)
entry.pack()
entry.bind("", launch_youtube)
root.mainloop()
Capture the video link with clipboard
If you do not want to copy and paste the url address adding this function and the button to activate the function
root1 = tk.Tk()
clip = root1.clipboard_get()
root1.withdraw()
e.set(clip)
print(clip)
root1.destroy()
The whole code
import tkinter as tk
import os, glob
root = tk.Tk()
lb = tk.Listbox(root)
lb.pack()
[lb.insert(0, f) for f in os.listdir() if f.endswith(".mp4")]
files = glob.glob("*.mp4")
def launch_mp4(event):
cur = lb.curselection()
get_filename = lb.get(cur)
os.startfile(get_filename)
def get_clipboard():
root1 = tk.Tk()
clip = root1.clipboard_get()
root1.withdraw()
e.set(clip)
print(clip)
root1.destroy()
lb.bind("<Double-Button>", launch_mp4)
def launch_youtube(event):
url = entry.get()
os.system(f"start {url}")
lab = tk.Label(root, text="Enter youtube url").pack()
e = tk.StringVar()
entry = tk.Entry(root, textvariable=e)
entry.pack()
entry.bind("<Return>", launch_youtube)
b_cnf = {
"text" : "Get clipboard",
"command": get_clipboard
}
b = tk.Button(cnf=b_cnf)
b.pack()
b2_cnf = {
"text" : "Launch",
"command": lambda : launch_youtube("event")
}
b2 = tk.Button(cnf=b2_cnf)
b2.pack()
root.mainloop()

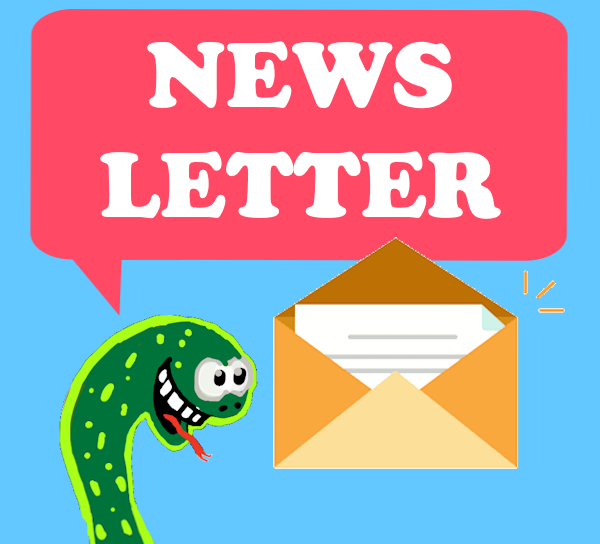


Twitter: @pythonprogrammi - python_pygame
Videos
Speech recognition gamePygame's Platform Game
