Module: pygame
To install pygame, simple open cmd and write:
pip install pygame
Objective: animate a sprite
We want to show an animated sprite witha a spreadsheet like the following image, i.e. a series of images that shown one after another give the illusion of movement. Alla the pictures are on the same file image (a png, for example). There are not 13 different files, but 1 png file with 13 different animations of the same 🦕, in this example. Let’s see how to show the animation correctly.

Load the spreadsheet
The images for the sprite can be downloaded here for free.

How to load a spreadsheet in pygame?
# load a spreadsheet import pygame import sys # main surface screen = pygame.display.set_mode((800, 600)) # surface 1 clock = pygame.time.Clock() img = pygame.image.load("img\\dino_1.png") nframes = 8 size = [int(img.get_width() / nframes), img.get_height()] print(size) animations = [] for x in range(nframes): frame_location = [size[0] * x, 0] # so starting with 0, x moves with each iteration 80 pxl to the right img_rect = pygame.Rect(frame_location, size) new_img = img.subsurface(img_rect) # not the same variable as img animations.append(new_img) print(animations) counter = 0 loop = 1 while loop: for event in pygame.event.get(): if event.type == pygame.QUIT: loop = 0 if counter < nframes -1: counter +=1 else: counter = 0 screen.fill((0,0,0)) screen.blit(animations[counter], (10, 150)) pygame.display.flip() clock.tick(10) pygame.quit() sys.exit()
How to join the images
In case you want to join the images with the different poses of the dino that are in different files, you can use this script below. Read this post about it and master it.
import numpy as np from PIL import Image import glob import sys print("To join vertically: py -m join v") print("To join horizzontally: py -m join h") def join(): list_im = glob.glob("*.png") imgs = [Image.open(i) for i in list_im] # resize images to the smallest one min_shape = sorted([(np.sum(i.size), i.size) for i in imgs])[0][1] imgs_comb = np.hstack((np.asarray(i.resize(min_shape)) for i in imgs)) # save that beautiful picture if sys.argv[1] == "v": # for a vertical stacking it is simple: use vstack imgs_comb = np.vstack((np.asarray(i.resize(min_shape)) for i in imgs)) imgs_comb = Image.fromarray(imgs_comb) imgs_comb.save('vertical.png') else: imgs_comb = Image.fromarray(imgs_comb) imgs_comb.save('horizontal.png') join()
Using a class
# load a spreadsheet import pygame import sys # main surface screen = pygame.display.set_mode((800, 600)) # surface 1 clock = pygame.time.Clock() class Animate(pygame.sprite.Sprite): def __init__(self, sheetname): super().__init__() self.sheetname = sheetname self.img = pygame.image.load(f"img\\{self.sheetname}.png") self.nframes = 8 self.size = [int(self.img.get_width() / self.nframes), self.img.get_height()] self.animations = [] for x in range(self.nframes): self.frame_location = [self.size[0] * x, 0] # so starting with 0, x moves with each iteration 80 pxl to the right self.img_rect = pygame.Rect(self.frame_location, self.size) self.new_img = self.img.subsurface(self.img_rect) # not the same variable as img self.animations.append(self.new_img) d = Animate("dino_1") counter = 0 loop = 1 while loop: for event in pygame.event.get(): if event.type == pygame.QUIT: loop = 0 if counter < d.nframes -1: counter +=1 else: counter = 0 screen.fill((0,0,0)) screen.blit(d.animations[counter], (10, 150)) pygame.display.flip() clock.tick(10) pygame.quit() sys.exit()
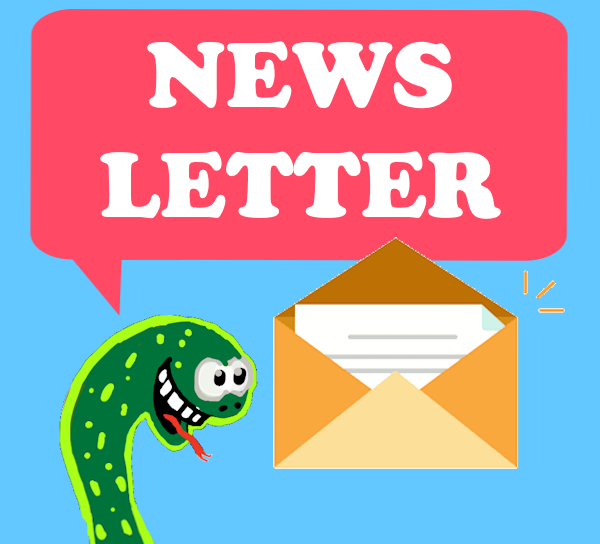


Twitter: @pythonprogrammi - python_pygame
Videos
Speech recognition gamePygame's Platform Game
