Again on windows in tkinter. Graphic user interface are funadamental to make things easy and fast even for people that do not know how to code. It’s also very useful for coders, to create utilities that speeds up their work. So, tkinter comes with Python and it’s very useful and easy to use after you spent some time experiment with it. It is one of the thing that is worth to learn.
Let’s make more windows from tkinter without using functions or classes, for semplicity.
This is the code.

This is the video tutorial.
This video has no audio, there is another video with the audio below.

import tkinter as tk root = tk.Tk() root.geometry("400x400") root.title("MAIN") text = tk.Text(root, font="Arial 26") text.pack() tl1 = tk.Toplevel(root) tl1.title("TL1") tl1.but1 = tk.Button(tl1, text="Click LT1 BT1", command=lambda: text.insert(tk.END, f"You clicked BT1 {tl1.but1['text']}")) tl1.but1.pack() tl2 = tk.Toplevel(root) tl2.title("TL2") root.mainloop()
If in this post here we’ve seen how to create many windows with tkinter, now we want to make it as easy as we can, without functions, so that you can immediately get what you have to do to open a new Window after you create the first one without having to care about anything else. In practice it is very simple, all you got to do is to use the Toplevel class. That’s it. To add buttons or other widget is just the same thing as for the main windows. Once you gave a name to the istance of Toplevel, you can add to it with a simple dot a name for a widget (in the example we called it tl1.but1, where tl stands for toplevel, so it’s clear that it’s a button of that window). The only difference among a widget of the main (root) window, of course, is that the first argument passed to the class Button of tkinter, will be tl1 because you want that button to appear on that toplevel window. So you can add the widgets you want and to show how you can interact among different windows, I also made happen that when you click on the button of the toplevel window n.1, something happens in the Text widget of the main window: some text appears. So you can manipulate stuffs in other window and you could use it to make some sort of submenu to do particular things in the main window (for example, if you want to make an app to draw on the main window, you could put on the toplevel window the button to erase the drawing, to choose another tool, increase the size of the pen etc.). Ok, so I think it’s all clear how to make more windows in the same one. Maybe in another post we could use a class to make our code more readable, who knows?
Another example of toplevel windows in tkinter with a button
Let’s make another simple example:
# the repository is here: https://github.com/formazione/tkinter_tutorial.git # GiovanniPython on YT # @pythonprogrammi on X import tkinter as tk from tkinter import messagebox root = tk.Tk() root.title("MAIN WINDOW") hello = tk.Toplevel(root) hello.title("Hello") hello.but1 = tk.Button(hello, text="Hello Button", command= lambda : messagebox.showinfo("Hello and attention", "A button in a secundary window has been pressed abruptely!") ) hello.but1.pack() root.mainloop()

In this case when we press the button on the toplevel window another window appear, this is made with messagebox.showinfo. We talked about this here, yes right here and here too.
See ya and keep improving your knowledge abut python and remember that to learn how to use it, the most important thing is to figure out on your own how to make things happen, because in a tutorial or in the documentation you can only find the fundamental aspects of the language, but it will be your problem solving ability to make it work for what you need. Find advices on blogs and stackoverflow to avoid inventing the wheel everytime, but many things, the best one comes from our own way of doing things. Another tip I want to give you is to split the problems into tiny steps and do always the simplest one at the beginning and then start to figure out how to implement this step into a more bigger plan.
Bye.
Video with audio
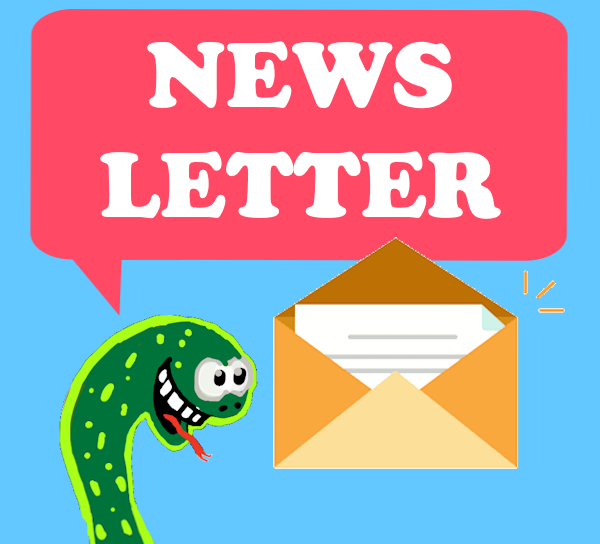


Twitter: @pythonprogrammi - python_pygame
Videos
Speech recognition gamePygame's Platform Game
