This is the way to see more tables or graphs in the same window with matplotlilb, the module for python to see data. In this case we take our code to analyse covid data about Italy, France and South Korea together. We have seen how to do it in other posts. Here I abstracted a little bit the code so that is easy to change data from one Country to another. I used subplot instead of tiledlayout to use it in this interactive shell on the webpage. Go to the link to get more informations about it. For what I experimented you just have to give subplot the number of tables you want in the same window as first argument and then 1 and then the number of order of the graph (1 for the first, 2 for the second ecc.).
Here is the code to embed it into a web page and run it from the web page itself with this codecamp shell. After the code, you will find the shell itself to see how it works.
<script async src="https://cdn.datacamp.com/dcl-react.js.gz"></script> <div class="exercise"> <div data-datacamp-exercise data-lang="python"> <code data-type="pre-exercise-code"> </code> <code data-type="sample-code"> import pandas as pd from datetime import datetime, timedelta import matplotlib.pyplot as plt def run(country, numtab): days = [] days2 = [] for n in range(5, 0, -1): day = datetime.today() - timedelta(n) days2.append(datetime(2020, day.month, day.day)) days.append(str(day.month) + "/" + str(day.day)) month = str(datetime.today().month) c = [] conf = 0 url = "https://raw.githubusercontent.com/CSSEGISandData/COVID-19/master/csse_covid_19_data/csse_covid_19_time_series/time_series_19-covid-Confirmed.csv" df = pd.read_csv(url, error_bad_lines=False) def check(day, n): global conf, what, x result = df.loc[df["Country/Region"]==country]["{}/20".format(day)] print(list(result)[0], end=" - ") if n == 0: perc = 0 if n > 0: diff = int(list(result)[0]) - x print("inc.: " + str(diff) + " ", end = "percent.: ") perc = int(diff / x * 100) print(int(diff / x * 100)) x = int(list(result)[0]) c.append(perc) for n,d in enumerate(days): print("{}".format(d), end=": ") check(d, n) print() print(c) #sorted(days, key=lambda d: map(int, d.split('/'))) def show(country): ax = plt.subplot(3,1,numtab) ax.bar(days2, c) ax.xaxis_date() plt.xlabel("days: ") plt.ylabel("Confirmed increment percentage") plt.title(country) plt.show() show(country) run("France", 1) run("Italy", 2) run("South Korea", 3) </code> <code data-type="solution"></code> <code data-type="sct"></code> <div data-type="hint">Just press 'Run'.</div> </div> </div>
Press RUN to see how it works
import pandas as pd
from datetime import datetime, timedelta
import matplotlib.pyplot as plt
def run(country, numtab):
days = []
days2 = []
for n in range(5, 0, -1):
day = datetime.today() - timedelta(n)
days2.append(datetime(2020, day.month, day.day))
days.append(str(day.month) + "/" + str(day.day))
month = str(datetime.today().month)
c = []
conf = 0
url = "https://raw.githubusercontent.com/CSSEGISandData/COVID-19/master/csse_covid_19_data/csse_covid_19_time_series/time_series_19-covid-Confirmed.csv"
df = pd.read_csv(url, error_bad_lines=False)
def check(day, n):
global conf, what, x
result = df.loc[df["Country/Region"]==country]["{}/20".format(day)]
print(list(result)[0], end=" - ")
if n == 0:
perc = 0
if n > 0:
diff = int(list(result)[0]) - x
print("inc.: " + str(diff) + " ", end = "percent.: ")
perc = int(diff / x * 100)
print(int(diff / x * 100))
x = int(list(result)[0])
c.append(perc)
for n,d in enumerate(days):
print("{}".format(d), end=": ")
check(d, n)
print()
print(c)
#sorted(days, key=lambda d: map(int, d.split('/')))
def show(country):
ax = plt.subplot(3,1,numtab)
ax.bar(days2, c)
ax.xaxis_date()
plt.xlabel("days: ")
plt.ylabel("Confirmed increment percentage")
plt.title(country)
plt.show()
show(country)
run("France", 1)
run("Italy", 2)
run("South Korea", 3)
For what we can see here, even if there are more confimed cases in South Korea, the increas is very low 8% or less, while in italy it’s around 25% and in France, that has less cases, it increases was in the last 2 day of 70% and 40% respectively.
Just the python code (without web page shell wrapper)
import pandas as pd from datetime import datetime, timedelta import matplotlib.pyplot as plt def run(country, numtab): days = [] days2 = [] for n in range(5, 0, -1): day = datetime.today() - timedelta(n) days2.append(datetime(2020, day.month, day.day)) days.append(str(day.month) + "/" + str(day.day)) month = str(datetime.today().month) c = [] conf = 0 url = "https://raw.githubusercontent.com/CSSEGISandData/COVID-19/master/csse_covid_19_data/csse_covid_19_time_series/time_series_19-covid-Confirmed.csv" df = pd.read_csv(url, error_bad_lines=False) def check(day, n): global conf, what, x result = df.loc[df["Country/Region"]==country]["{}/20".format(day)] print(list(result)[0], end=" - ") if n == 0: perc = 0 if n > 0: diff = int(list(result)[0]) - x print("inc.: " + str(diff) + " ", end = "percent.: ") perc = int(diff / x * 100) print(int(diff / x * 100)) x = int(list(result)[0]) c.append(perc) for n,d in enumerate(days): print("{}".format(d), end=": ") check(d, n) print() print(c) #sorted(days, key=lambda d: map(int, d.split('/'))) def show(country): ax = plt.subplot(3,1,numtab) ax.bar(days2, c) ax.xaxis_date() plt.xlabel("days: ") plt.ylabel("Confirmed increment percentage") plt.title(country) plt.show() show(country) run("France", 1) run("Italy", 2) run("South Korea", 3)
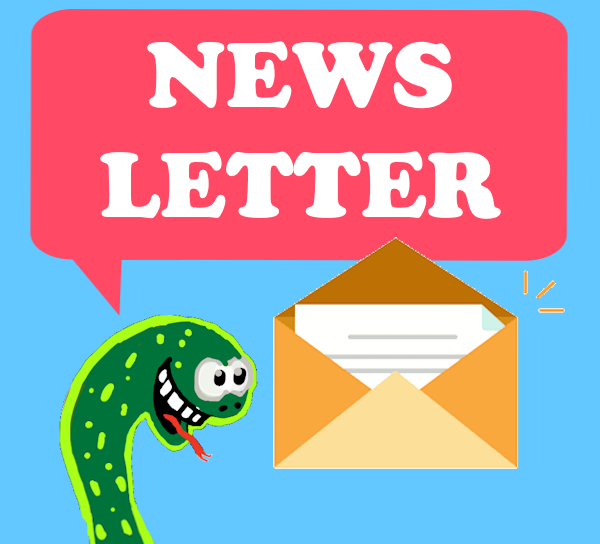


Twitter: @pythonprogrammi - python_pygame
Videos
Speech recognition gamePygame's Platform Game
