A script to open images with a button in the pygame window. The images is shown into the window.

import tkinter as tk from tkinter import filedialog import button as bt # Hello, this is a snippet import pygame pygame.init() screen = pygame.display.set_mode((800, 600)) pygame.display.set_caption('Quick Start') clock = pygame.time.Clock() background = pygame.Surface((800, 600)) background.fill(pygame.Color('#000000')) images = pygame.sprite.Group() class Image(pygame.sprite.Sprite): def __init__(self, file_path, x, y): super().__init__() self.image = pygame.image.load(file_path) self.rect = self.image.get_rect() self.rect.x = x self.rect.y = y images.add(self) def openf(): file_path = filedialog.askopenfilename() # img = tk.PhotoImage(file=self.file_path) image = Image(file_path, 0, 30) b1 = bt.Button() b1.render("Open_file") b1.command = openf is_running = True while is_running: screen.blit(background, (0, 0)) for event in pygame.event.get(): if event.type == pygame.QUIT: is_running = False images.update() images.draw(screen) bt.buttons.update() bt.buttons.draw(screen) pygame.display.update() clock.tick(60) pygame.quit()
The button script imported by the main file
import pygame screen = pygame.display.set_mode((800, 600)) buttons = pygame.sprite.Group() class Button(pygame.sprite.Sprite): ''' Create a button clickable with changing hover color''' def __init__(self, position=(0,0), text="Click", size=16, colors="white on blue", hover_colors="red on green", style=1, borderc=(255,255,255), command=lambda: print("No command activated for this button")): # the hover_colors attribute needs to be fixed super().__init__() self.text = text self.command = command # --- colors --- self.colors = colors self.original_colors = colors self.fg, self.bg = self.colors.split(" on ") if hover_colors == "red on green": self.hover_colors = f"{self.bg} on {self.fg}" else: self.hover_colors = hover_colors self.style = style self.borderc = borderc # for the style2 # font self.font = pygame.font.SysFont("Arial", size) self.position = position self.render(self.text) buttons.add(self) def render(self, text): self.text = text self.image = self.font.render(self.text, 1, self.fg) self.x, self.y, self.w , self.h = self.image.get_rect() self.x, self.y = self.position self.rect = pygame.Rect(self.x, self.y, self.w, self.h) self.pressed = 1 ''' create the surface with a text in self.image ''' self.rect = self.image.get_rect() def update(self): ''' called by buttons.update, the group of buttons ''' self.fg, self.bg = self.colors.split(" on ") if self.style == 1: self.draw_button1() elif self.style == 2: self.draw_button2() self.hover() self.click() def draw_button1(self): ''' draws 4 lines around the button and the background ''' # horizontal up pygame.draw.line(screen, (150, 150, 150), (self.x, self.y), (self.x + self.w , self.y), 5) pygame.draw.line(screen, (150, 150, 150), (self.x, self.y - 2), (self.x, self.y + self.h), 5) # horizontal down pygame.draw.line(screen, (50, 50, 50), (self.x, self.y + self.h), (self.x + self.w , self.y + self.h), 5) pygame.draw.line(screen, (50, 50, 50), (self.x + self.w , self.y + self.h), [self.x + self.w , self.y], 5) # background of the button pygame.draw.rect(screen, self.bg, (self.x, self.y, self.w , self.h)) def draw_button2(self): ''' a linear border ''' pygame.draw.rect(screen, self.bg, (self.x, self.y, self.w , self.h)) pygame.gfxdraw.rectangle(screen, (self.x, self.y, self.w , self.h), self.borderc) def hover(self): ''' checks if the mouse is over the button and changes the color if it is true ''' if self.rect.collidepoint(pygame.mouse.get_pos()): # you can change the colors when the pointer is on the button if you want self.colors = self.hover_colors # pygame.mouse.set_cursor(*pygame.cursors.diamond) else: self.colors = self.original_colors self.render(self.text) def click(self): ''' checks if you click on the button and makes the call to the action just one time''' if self.rect.collidepoint(pygame.mouse.get_pos()): if pygame.mouse.get_pressed()[0] and self.pressed == 1: print("Execunting code for button '" + self.text + "'") self.command() self.pressed = 0 if pygame.mouse.get_pressed() == (0,0,0): self.pressed = 1
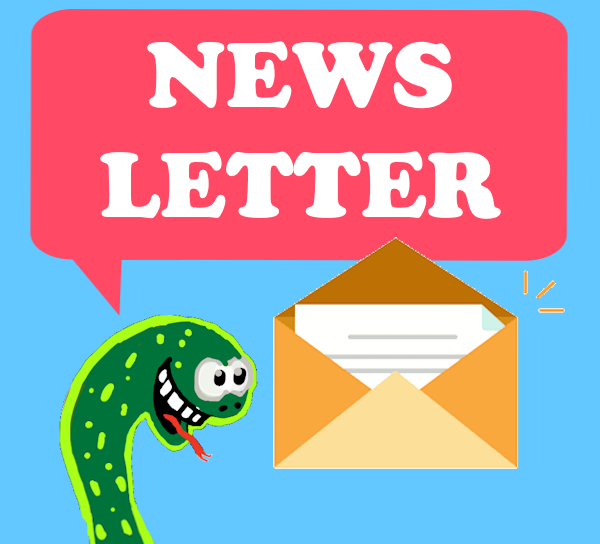


Twitter: @pythonprogrammi - python_pygame
Videos
Speech recognition gamePygame's Platform Game
