In the last post I showed you how to send different emails to different addresses with python. This time we are going a little further showing a practical example of a test with different data sent to different students and having the solution save in a file, so that we ca easily correct the different tests, without having to stress out too much having different solutions for each test. This is the code and it should be watched after seeing the last post.
The code to send the exercises
import smtplib from credentials import * from random import choice # THE STUDENTS EMAILS receivers = [ "[email protected]", "[email protected]", "[email protected]"] # RANDOM DATA FOR THE TEST messages = ['[email protected]\n==========\n\nCreate a balance sheet and income statement based on these data:\n\nOperating costs 40000\ncurrent assets 16000\nassets 40000\ntaxes 2700\nrevenues 50000\nfinancial charges 1000\ndebts 22400\n\n\nPut the voices in the balance sheet and income statement.\nFind the net assets and the economic result\n\n', '[email protected]\n==========\n\nCreate a balance sheet and income statement based on these data:\n\nOperating costs 8000\ncurrent assets 16000\nassets 40000\ntaxes 300\nrevenues 10000\nfinancial charges 1000\ndebts 22400\n\n\nPut the voices in the balance sheet and income statement.\nFind the net assets and the economic result\n\n', '[email protected]\n==========\n\nCreate a balance sheet and income statement based on these data:\n\nOperating costs 24000\ncurrent assets 16000\nassets 40000\ntaxes 1200\nrevenues 30000\nfinancial charges 2000\ndebts 22400\n\n\nPut the voices in the balance sheet and income statement.\nFind the net assets and the economic result\n\n'] # ASSIGN RANDOM DATA TO message mail = username # il mittente def different_email(): for n, msg in enumerate(messages): server = smtplib.SMTP("smtp.gmail.com", 587) server.ehlo() server.starttls() server.ehlo() server.login(username, password) server.sendmail( mail, receivers[n], messages[n]) server.quit() different_email()
The credentials.py file
You need to create a file with your credentials. It is very easy. I explained this part here.
The file to make the text for the different tets
So, you need this second file. You do this. Copy the text for the array of tests into the previous one and you are good to go. In a next post I will make some more consistent code to make this work with just one script and, maybe, even with a grphic user interface, using tkinter. Until then, here is our code. Do not worry, it’s all explained in the video below, anyway.
from random import choice import os text = """ Create a balance sheet and income statement based on these data: Operating costs {} current assets {} assets {} taxes {} revenues {} financial charges {} debts {} Put the voices in the balance sheet and income statement. Find the net assets and the economic result """ students = [ "[email protected]", "[email protected]", "[email protected]" ] t = [] sol = [] for m in students: rev = choice([10000, 20000, 30000, 40000, 50000]) opcosts = rev // 10 * 8 assets = choice([50000, 40000, 60000]) curas = assets // 10 * 4 fin = choice([800, 700, 600, 1000, 2000]) debts = (assets + curas) // 10 * 4 taxes = (rev - opcosts - fin) // 10 * 3 rnddata = [ opcosts, curas, assets, taxes, rev, fin, debts ] text2 = text.format(*rnddata) singlex = m + "\n==========\n" + text2 t.append(singlex) s = "net assets: " + str(assets + curas - debts) s += "\n" s += "economic result: " + str(rev - opcosts - fin - taxes) sol.append(singlex + s) print(t) print("SOLUTIONS") print(*sol, sep="\n") with open("solutions.txt", "w", encoding="utf-8") as file: file.write(sol)
The video: make differents test to send by email to students in seconds
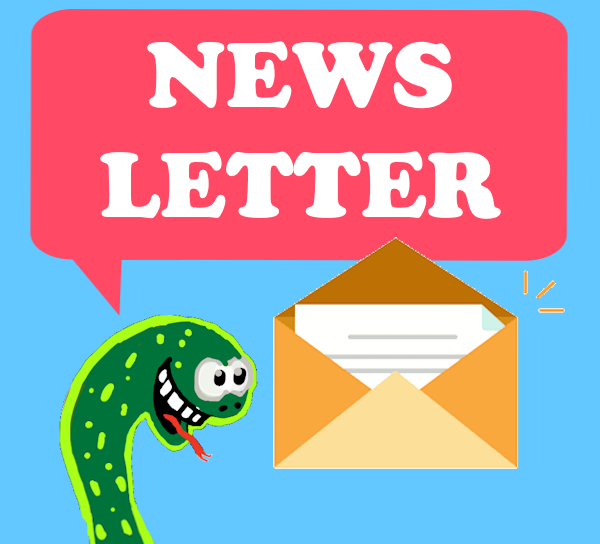


Twitter: @pythonprogrammi - python_pygame
Videos
Speech recognition gamePygame's Platform Game
