I am updating the pygame_quiz project, adding the chance to change the color to the label widget.
So I’ve:
- added color to label
- added a sound when you click on a button
You can find the code here
https://github.com/formazione/pygame_quiz

The Label class
The labels list will contain the istances of the class so that we can show them easily in the while loop of the ‘game’.
labels = [] class Label: ''' CLASS FOR TEXT LABELS ON THE WIN SCREEN SURFACE ''' def __init__(self, screen, text, x, y, size=20, color="white"): if size != 20: self.font = fontsize(size) else: self.font = font_default self.image = self.font.render(text, 1, color) _, _, w, h = self.image.get_rect() self.rect = pygame.Rect(x, y, w, h) self.screen = screen labels.append(self) def change_text(self, newtext, color="white"): self.image = self.font.render(newtext, 1, color) def draw(self): self.screen.blit(self.image, (self.rect))
This is the function to be called in the while loop that will show the labels
def show_labels(): for _ in labels: _.draw()
To create some istances you can go like this
score = Label(screen, “Punteggio”, 100, 300)
Every label will go in the list and when you write the loop for the window to interact with the user, you call the function show_labels (you can also write directly the for loop of this function in the while loop if you want).
if __name__ == '__main__': pygame.init() game_on = 0 def loop(): # BUTTONS ISTANCES game_on = 1 question(qnum) while True: screen.fill(0) for event in pygame.event.get(): if (event.type == pygame.QUIT): game_on = 0 pygame.quit() if event.type == pygame.KEYDOWN: if event.key == pygame.K_ESCAPE: pygame.quit() game_on = 0 if game_on: buttons.update() buttons.draw(screen) else: pygame.quit() sys.exit() show_labels() # =================== THIS SHOWS THE LABELS clock.tick(60) pygame.display.update() pygame.quit()
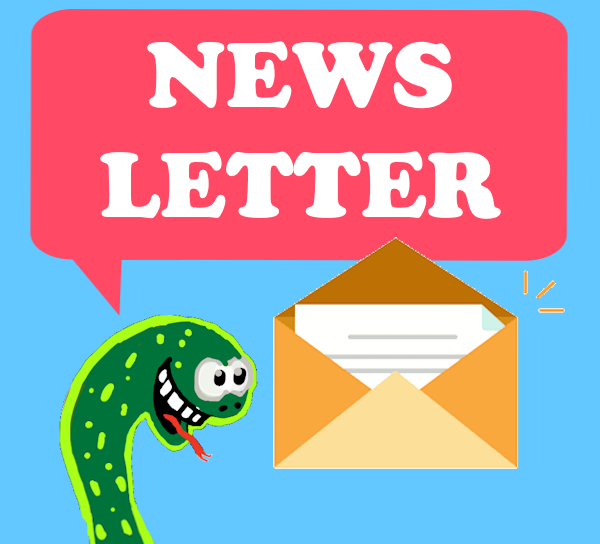


Twitter: @pythonprogrammi - python_pygame
Videos
Speech recognition gamePygame's Platform Game
