Today we are going to see how to animate sprites
import pygame import glob class MySprite(pygame.sprite.Sprite): def __init__(self): super(MySprite, self).__init__() self.images = [] # === to put the image in order === 1, 2, 3.... 9, 10, 11 # otherwise it would go 1, 11, 12, ... 2, 3 self.imageslist = glob.glob("png\\*.png") self.idle = self.get_images("Idle") self.walk = self.get_images("Walk") self.jump = self.get_images("Jump") print(self.idle) self.index = 0 self.rect = pygame.Rect(5, 5, 150, 198) self.state = 0 self.images = self.idle # my_group.add(self) def get_images(self, name): ''' give the starting of the name of animation to load the images for that action to add an animation images list: self.shoot = get_images('Shoot') * all images must start with Shoot and end with a progressive number in folder png if you want to animate enemies, give the images the name enemy1Idle 1 ..., enemy1Walk 1... etc enemy2Idle1... ''' temp = [i for i in self.imageslist if i.startswith(f"png\\{name}")] first = len(temp[0]) idle1 = [] idle2 = [] for i in temp: if len(i) == first: idle1.append(i) else: idle2.append(i) temp = idle1 + idle2 # now the order should be correct temp = [pygame.image.load(i).convert() for i in temp] return temp def user_interaction(self): ''' called by update of this class ''' for event in pygame.event.get(): if event.type == pygame.QUIT: pygame.quit() if event.type == pygame.KEYDOWN: # IF YOU PRESS RIGHT PLAYER WALKS (see update method) if event.key == pygame.K_RIGHT: self.state = 1 # IF YOU PRESS UP PLAYER JUMPS (see update method) if event.key == pygame.K_UP: self.state = 2 # WHEN YOU DO not HOLD key it goes back to IDLE if event.type == pygame.KEYUP: self.state = 0 def update(self): # CHECK KEY PRESSED self.user_interaction() # LOADS THE ANIMATION FOR THE ACTION AS YOU PRESS A KEY ''' -------------------- state 0 idle 1 walk 2 jump --------------------- ''' if self.state == 0: self.images = self.idle # YOU PRESS RIGHT ARROW: WALKS elif self.state == 1: self.images = self.walk # YOU PRESS UP, JUMPS elif self.state == 2: self.images = self.jump # ITERATE THROUGH IMAGES FOR ANIMATION if int(self.index) >= len(self.images): self.index = 0 self.image = self.images[int(self.index)] self.index += .3 def show_fps(): ''' shows the frame rate on the screen ''' fps_text = str(int(clock.get_fps())) # get the clocl'fps # render a text surface fps_surface = fps_font.render(fps_text, 1, pygame.Color("black")) # blit the background surface screen.blit(fps_bg, (0, 0)) # blit the text surface on the backgroud screen.blit(fps_surface, (0, 0)) pygame.init() SIZE = WIDTH, HEIGHT = 600, 550 #the width and height of our screen FPS = 60 #Frames per second fps_bg = pygame.Surface((20, 25)) # a surface for fps fps_bg.fill((255, 255, 255)) # the color of the surface fps_font = pygame.font.SysFont("Arial", 20) # a font for the fps clock = pygame.time.Clock() screen = pygame.display.set_mode(SIZE) def main(): pygame.display.set_caption("Trace") player = MySprite() my_group = pygame.sprite.Group(player) while True: # to get the users events see self.user_interaction in MySprite my_group.update() # screen.fill((0,0,0)) my_group.draw(screen) show_fps() pygame.display.update() clock.tick(FPS) pygame.quit() if __name__ == '__main__': main()
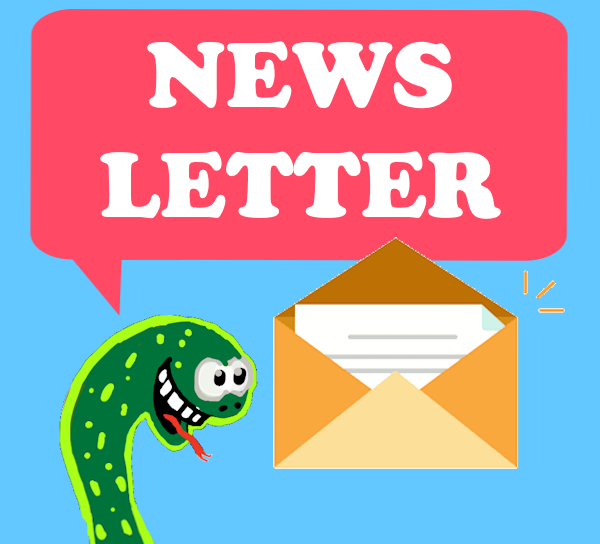


Twitter: @pythonprogrammi - python_pygame
Videos
Speech recognition gamePygame's Platform Game
