This time we start with making a bullet sprite for the enemy, so that he can shoot at us. This is the first part, we will just place the bullet and make it visible, so that we understand what we’re doing. We also make the bullet point towards the player.
This is the new class for the bullet
class Bullet(): def __init__(self): self.x = enemy.x self.y = enemy.y self.image = pygame.image.load("img/bullet.png").convert() self.rect = self.image.get_rect() def draw(self): if enemy.x < player.x: self.rect[0] = enemy.x + 50 if enemy.x > player.x: self.rect[0] = enemy.x if abs(enemy.x - player.x) < 8: self.rect[0] = enemy.x + 24 if enemy.y > player.y: self.rect[1] = enemy.y if enemy.y < player.y: self.rect[1] = enemy.y + 50 if abs(enemy.y - player.y) < 8: self.rect[1] = enemy.y + 24 screen.blit(self.image, self.rect)
This is the instance fo the 3 sprites now
player = Player(0,0) enemy = Enemy(100,100) bullet = Bullet()
in the mainloop nethod of the Screen class we show the bullet like this, with bullet.draw
Screen.check_collision() screen.fill(0) show_fps() player.draw() enemy.draw() bullet.draw() enemy.move_ai() pygame.display.update() clock.tick(60)
So to create a bullet we:
- created a class Bullet
- made an istance of Bullet called bullet
- call every frame the method bullet.draw to show it
- in this method we compare the position of player and enemy to position the bullet
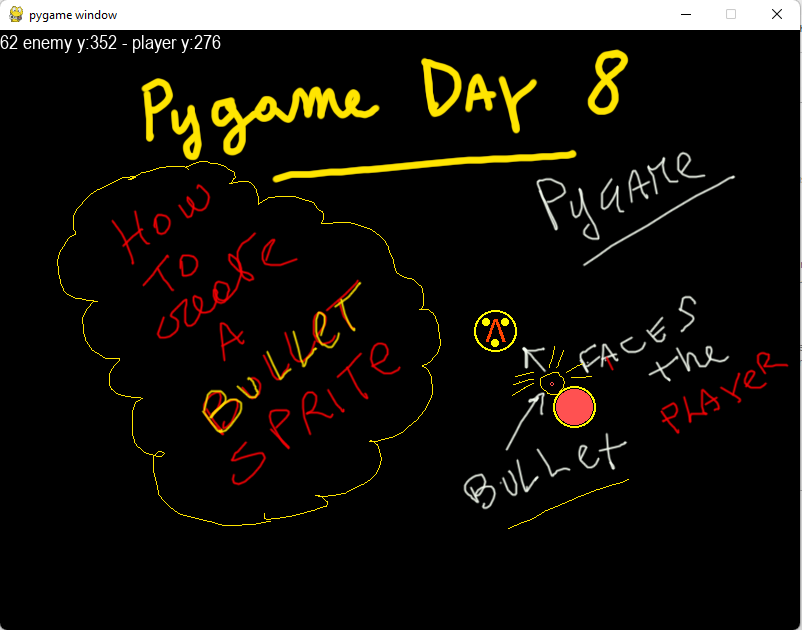
https://github.com/formazione/pygame_days
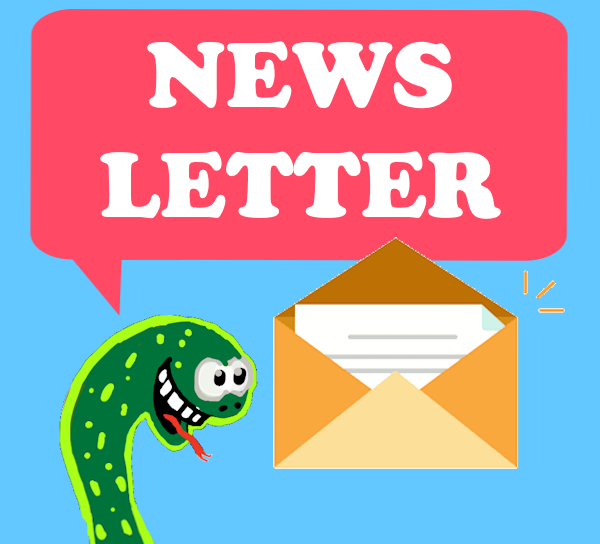


Twitter: @pythonprogrammi - python_pygame
Videos
Speech recognition gamePygame's Platform Game
