
For the script of the day we got a walking cat.
import pygame from glob import glob import sys pygame.init() screen = pygame.display.set_mode((400, 600)) def debug(message="ended"): print("\n", message, "\n") pygame.quit() sys.exit() class Cat: def __init__(self, x, y): self.x = x self.y = y # list of surfaces with all the images for the different animatios self.idle = [pygame.image.load(f).convert_alpha() for f in glob(f"cat/Idle*.png")[1:]] self.walk = [pygame.image.load(f).convert_alpha() for f in glob(f"cat/Walk*.png")[1:]] self.imagelist = self.idle print(self.imagelist) # debug() # starting animation self.images_counter = 0 self.image = self.imagelist[0] self.direct = "" self.store_direction = "" def update(self): self.images_counter += .4 if self.images_counter >= len(self.imagelist): #debug(len(self.imagelist)) self.images_counter = 0 if self.direct == "right": self.imagelist = self.walk self.image = self.imagelist[int(self.images_counter)] self.store_direction = "right" screen.blit(cat.image, (cat.x, cat.y)) elif self.direct == "left": self.imagelist = self.walk self.image = self.imagelist[int(self.images_counter)] screen.blit(pygame.transform.flip(cat.image, True, False), (cat.x, cat.y)) self.store_direction = "left" else: self.imagelist = self.idle self.image = self.imagelist[int(self.images_counter)] if self.store_direction == "left": screen.blit(pygame.transform.flip(cat.image, True, False), (cat.x, cat.y)) else: screen.blit(cat.image, (cat.x, cat.y)) cat = Cat(100, 100) square = pygame.Surface((cat.image.get_size())) square.fill((0, 180, 250)) sw, sh = screen.get_size() clock = pygame.time.Clock() while True: screen.fill((128, 255, 128)) # screen.blit(pygame.transform.scale(cat, (sw, sh)),(0, 0)) #screen.blit(square, (Cat.x, Cat.y)) match cat.direct: case "right": cat.x += 2 case "left": cat.x -= 2 if pygame.event.get(pygame.QUIT): break for event in pygame.event.get(): if event.type == pygame.KEYDOWN: if event.key == pygame.K_RIGHT: cat.direct = "right" if event.key == pygame.K_LEFT: cat.direct = "left" if event.key == pygame.K_UP: cat.y -= 10 if event.key == pygame.K_DOWN: cat.y += 10 if event.type == pygame.KEYUP: cat.direct = "" cat.update() pygame.display.update() clock.tick(60) pygame.quit()
My code in Internet (thanks to pygbag)
Pygame in the browser? Is it possible? What is this revolution? Yes, it can sound incredible, but thanks to Wasm and the fact that it supports SDL2 that by the way is what pygame uses, we got this incredible change to use and make people use pygame, our apps, into the browser, simply giving them the link, not having to install anything. This is one of the most exciting thing of the latest time aroung pygame and python too, among other great news (more speed, python integrated in excel. facebook working to improve python for the future etc.). So, now you can make games that can become viral, it’s a reason to start learning or improving your pygame skill. Com’on the time is now and only who starts first can make the difference. Who came later, well… too late. So this will be the year of pygame videogame invasion. Are you ready?

https://formazione.github.io/cat/index.html
The following code is the one that you can see going to the code above and it’s always into the repository. If you want to see how to use pygbag to see pygame into the browser and make it playable from the browser by anyone without installing anything read this post about pygabag and pygame in the browser.
import pygame from glob import glob import sys from pygame.locals import * pygame.init() WINDOWWIDTH = w = 400 WINDOWHEIGHT = h = 400 screen = pygame.display.set_mode((w, h)) class Sprite(pygame.sprite.Sprite): def __init__(self, x, y): super(Sprite, self).__init__() self.x = x self.y = y self.dogwalking = glob("dog/Walk*.png") self.dogidling = glob("dog/Idle*.png") self.load_images() def load(self, x): return pygame.image.load(x).convert_alpha() def flip(self, x): return pygame.transform.flip(self.load(x), 1, 0) def load_images(self): self.list = [self.load(f) for f in self.dogwalking] self.listflip = [self.flip(f) for f in self.dogwalking] self.list_idle = [self.load(f) for f in self.dogidling] self.list_idleflip = [self.flip(f) for f in self.dogidling] self.counter = 0 self.image = self.list[0] self.rect = self.image.get_rect() self.dir = "" self.prov = "" g.add(self) def update_counter(self, vel, img_list): self.counter += vel if self.counter >= len(img_list): self.counter = 0 self.image = img_list[int(self.counter)] def update(self): if moveRight: self.update_counter(.1, self.list) self.prov = self.dir if moveLeft: self.update_counter(.1, self.listflip) # self.image = self.listflip[int(self.counter)] self.prov = self.dir if self.dir == "": self.update_counter(.1, self.list_idle) if moveRight: self.image = self.list_idle[int(self.counter)] else: self.image = self.list_idleflip[int(self.counter)] g = pygame.sprite.Group() player = Sprite(100, 100) clock = pygame.time.Clock() moveLeft = False moveRight = False moveUp = False moveDown = False MOVESPEED = 1 while True: # Check for events. for event in pygame.event.get(): if event.type == QUIT: pygame.quit() sys.exit() if event.type == KEYDOWN: # Change the keyboard variables. if event.key == K_LEFT or event.key == K_a: moveRight = False moveLeft = True if event.key == K_RIGHT or event.key == K_d: moveLeft = False moveRight = True player.image = player.list[int(player.counter)] if event.key == K_UP or event.key == K_w: moveDown = False moveUp = True if event.key == K_DOWN or event.key == K_s: moveUp = False moveDown = True # KEYUP if event.type == KEYUP: player.counter = 0 if event.key == K_ESCAPE: pygame.quit() sys.exit() if event.key == K_LEFT or event.key == K_a: moveLeft = False if event.key == K_RIGHT or event.key == K_d: moveRight = False if event.key == K_UP or event.key == K_w: moveUp = False if event.key == K_DOWN or event.key == K_s: moveDown = False # Draw the white background onto the surface. screen.fill((255, 255, 255)) # Move the player. if moveDown and player.rect.bottom < WINDOWHEIGHT: player.rect.top += MOVESPEED if moveUp and player.rect.top > 0: player.rect.top -= MOVESPEED if moveLeft and player.rect.left > -35: player.rect.left -= MOVESPEED try: player.counter += .1 player.image = pygame.transform.flip(player.list[int(player.counter)], True, False) except: player.counter = 0 player.image = pygame.transform.flip(player.list[int(player.counter)], True, False) if moveRight and player.rect.right < WINDOWWIDTH + 35: player.rect.right += MOVESPEED try: player.counter -= .1 player.image = player.list[int(player.counter)] except: player.counter = 0 player.image = player.list[int(player.counter)] # Draw the player onto the surface. g.draw(screen) g.update() # Draw the window onto the screen. pygame.display.update() clock.tick(120) pygame.quit()
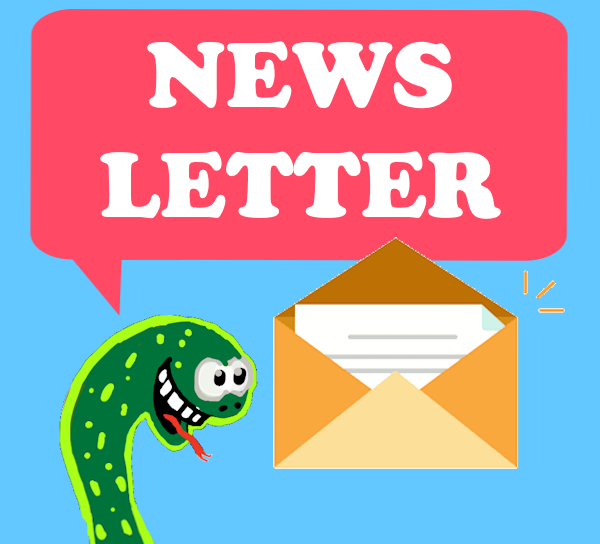


Twitter: @pythonprogrammi - python_pygame
Videos
Speech recognition gamePygame's Platform Game
