Improvising the code to make a calculator from scratch. I made this first part of the code and there is the video of the making. This is the first part, that will allow you to make just sums. I will not use eval, but just regular python operations with variables. Let’s see the code and the video here in the following part of the post.
This is the output, by the way. In the next video the final part.

The code of the first part
This code makes only sums…
import tkinter as tk import tkinter.ttk as ttk counter = 0 crow = 0 mem1 = 0 def setEntry(value): global esv global mem1 v = esv.get() v += value esv.set(v) if value == "+": mem1 = v.replace("+", "") esv.set("") if value == "=": mem2 = v.replace("=","") mem1 = int(mem1) + int(mem2) esv.set(mem1) def Button(text): global counter global crow global entry global esv b = ttk.Button(root, text=text) root.columnconfigure(counter, weight=1) b["command"] = lambda: setEntry(b["text"]) b.grid(row=1+crow, column=counter, sticky="nswe",ipady=10, ipadx=10) counter += 1 if counter > 2: crow +=1 counter = 0 return b def main(): global root global esv root = tk.Tk() root.title("CalpPy") esv = tk.StringVar() entry = tk.Entry(root, textvariable=esv, justify="right") entry["font"] = "arial 24" entry["bg"] = "cyan" entry.grid(row=0, column=0, columnspan=3, sticky="nswe") # Buttons for but in [7, 8, 9, 4, 5, 6, 1, 2, 3, 0, "+", "-", "x", ":", "="]: b = Button(str(but)) root.mainloop() main()
The live coding video to make a calculator part 1
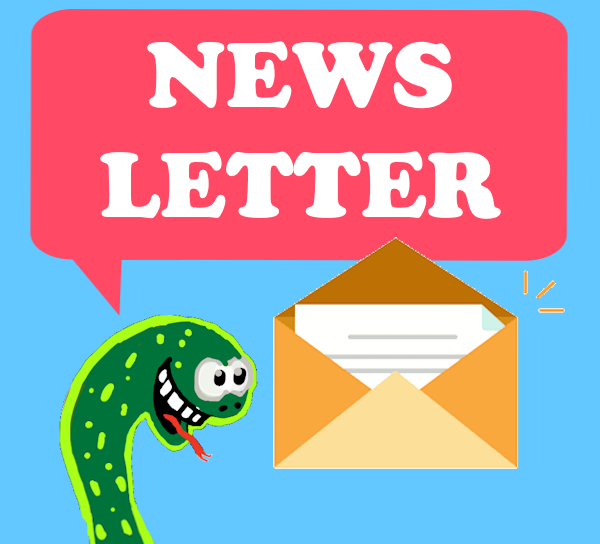


Twitter: @pythonprogrammi - python_pygame
Videos
Speech recognition gamePygame's Platform Game
