To send an attachment in an email you need to (take from here):
- Create MIME
- Add sender, receiver address into the MIME
- Add the mail title into the MIME
- Attach the body into the MIME
- Open the file as binary mode, which is going to be attached with the mail
- Read the byte stream and encode the attachment using base64 encoding scheme.
- Add header for the attachments
- Start the SMTP session with valid port number with proper security features.
- Login to the system.
- Send mail and exit
The modules
These are the modules needed
import smtplib from email.mime.multipart import MIMEMultipart from email.mime.text import MIMEText from email.mime.base import MIMEBase from email import encoders
What’s a MIME?
The MIME (Multipurpose Internet Mail Extensions) is an Internet standard that extends the format of email messages to support text in character sets other than ASCII, as well as attachments of audio, video, images, and application programs.
The body of the email
body = '''Hello, This is the body of the email sicerely yours G.G. '''
Sender and receiver
sender = '[email protected]' password = 'xxxxxxxx' receiver = '[email protected]'
Let’s setup the MIME
#Setup the MIME message = MIMEMultipart() message['From'] = sender message['To'] = receiver message['Subject'] = 'This email has an attacment, a pdf file'
We attach the body (MIMEText)
message.attach(MIMEText(body, 'plain'))
Attach the pdf
pdfname = 'TP_python_prev.pdf' # open the file in bynary binary_pdf = open(pdfname, 'rb') payload = MIMEBase('application', 'octate-stream', Name=pdfname) payload.set_payload((binary_pdf).read()) # enconding the binary into base64 encoders.encode_base64(payload) # add header with pdf name payload.add_header('Content-Decomposition', 'attachment', filename=pdfname) message.attach(payload)
Create Smtp session to send email
#use gmail with port session = smtplib.SMTP('smtp.gmail.com', 587) #enable security session.starttls() #login with mail_id and password session.login(sender, password) text = message.as_string() session.sendmail(sender, receiver, text) session.quit() print('Mail Sent')
The whole code
import smtplib from email.mime.multipart import MIMEMultipart from email.mime.text import MIMEText from email.mime.base import MIMEBase from email import encoders body = '''Hello, This is the body of the email sicerely yours G.G. ''' # put your email here sender = '[email protected]' # get the password in the gmail (manage your google account, click on the avatar on the right) # then go to security (right) and app password (center) # insert the password and then choose mail and this computer and then generate # copy the password generated here password = 'xxxxxxxxxxxxxxxxxxxxxxxx' # put the email of the receiver here receiver = '[email protected]' #Setup the MIME message = MIMEMultipart() message['From'] = sender message['To'] = receiver message['Subject'] = 'This email has an attacment, a pdf file' message.attach(MIMEText(body, 'plain')) pdfname = 'es_full.pdf' # open the file in bynary binary_pdf = open(pdfname, 'rb') payload = MIMEBase('application', 'octate-stream', Name=pdfname) # payload = MIMEBase('application', 'pdf', Name=pdfname) payload.set_payload((binary_pdf).read()) # enconding the binary into base64 encoders.encode_base64(payload) # add header with pdf name payload.add_header('Content-Decomposition', 'attachment', filename=pdfname) message.attach(payload) #use gmail with port session = smtplib.SMTP('smtp.gmail.com', 587) #enable security session.starttls() #login with mail_id and password session.login(sender, password) text = message.as_string() session.sendmail(sender, receiver, text) session.quit() print('Mail Sent')
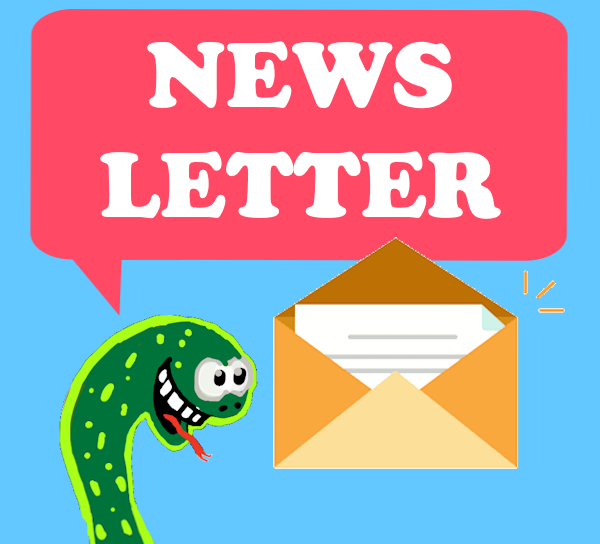


Twitter: @pythonprogrammi - python_pygame
Videos
Speech recognition gamePygame's Platform Game
