Let’s make a very simple todo app.
The app will ask you what you want to add to a todo.txt file and then you write it in the cmd and it will append your response in the file that will open up showing what’s inside. If you use Windows the notepad will open the text file called todo.
It will also add the date of your note, so that you can know when you added it, without having to write it manually.
The today function
The function for the date is this:
def today(): oggi = str(datetime.today()).split(" ")[0] y,m,d = oggi.split("-") return f"{d}/{m}/{y}"
It uses datetime.today (you got to import datetime from datetime, as you will see in the full code example at the end of this post). It splits the string returned by this function getting the first one where there is the year, month ad day, it splits the 3 data in memory places named y, m and d and then returns the date in a european form. You can modify the order of d/m/y as the one used in your country changing the order of the return statement in the function.
The write function
This one opens the file in append mode, so that everytime you run the script it will add the item to the todo list without deleting the previous ones as it would happen if you put “w” instead of “a”.
from datetime import datetime import os def today(): oggi = str(datetime.today()).split(" ")[0] y,m,d = oggi.split("-") return f"{d}/{m}/{y}" def write(file, text): print(os.getcwd()) with open(file, "a") as f: print(text, file=f) os.startfile(file) if __name__ == "__main__": todo = input("Add to todo> ") write("todo.txt", today() + " " + todo)
That’s all. You could imagine creating a tkinter GUI for this, using this functions into the GUI. See ya next time.

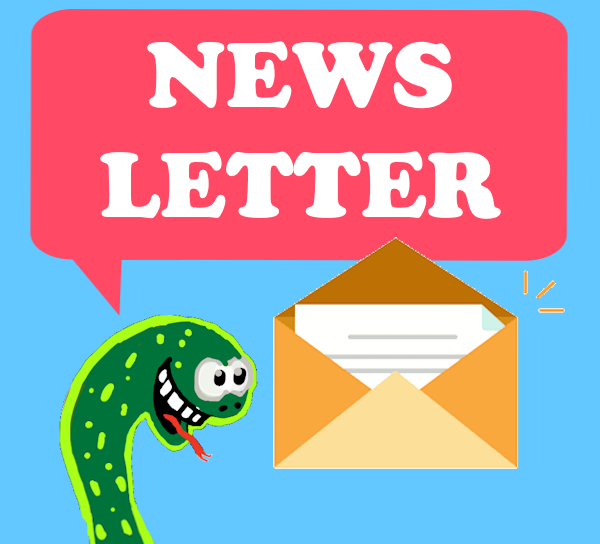


Twitter: @pythonprogrammi - python_pygame
Videos
Speech recognition gamePygame's Platform Game
