This script makes you speak the pc
from gtts import gTTS from io import BytesIO import pygame import time def wait(): while pygame.mixer.get_busy(): time.sleep(1) def speak(text, language='en'): ''' speaks without saving the audio file ''' mp3_fo = BytesIO() tts = gTTS(text, lang=language) tts.write_to_fp(mp3_fo) mp3_fo.seek(0) sound = pygame.mixer.Sound(mp3_fo) sound.play() wait() def speaksave(text, language='en'): ''' saves the audio file and then speaks ''' tts = gTTS(text, lang=language) tts.save("myfile.mp3") sound = pygame.mixer.Sound("myfile.mp3") sound.play() wait() pygame.init() pygame.mixer.init() speaksave("This audio is saved as myfile.mp3, look in the folder") speak("This audio is not saved")
Let’s make some more choices
Here you can choose to hear the words without saving them, saving them in different files, one for each row, or saving all the text in one audio file.
from gtts import gTTS from io import BytesIO import pygame import time def wait(): while pygame.mixer.get_busy(): time.sleep(.1) def process_speak(text="", language='en'): ''' speaks without saving the audio file ''' wait() mp3_fo = BytesIO() tts = gTTS(text, lang=language) tts.write_to_fp(mp3_fo) mp3_fo.seek(0) sound = pygame.mixer.Sound(mp3_fo) sound.play() wait() def save_(name="myfile", text="", language='en'): ''' saves the audio file and then speaks ''' global filecount tts = gTTS(text, lang=language) tts.save(f"{name}{filecount}.mp3") sound = pygame.mixer.Sound(f"{name}{filecount}.mp3") filecount+=1 sound.play() wait() def save_file(name="myfile", text="", language='en'): ''' saves the audio file and then speaks ''' global filecount text = ",".join(text) tts = gTTS(text, lang=language) tts.save(f"{name}{filecount}.mp3") sound = pygame.mixer.Sound(f"{name}{filecount}.mp3") sound.play() wait() def speak(text: list or str): ''' use speak as many lines are there into text ''' if type(text) == list: for t in text: process_speak(t) elif type(text) == str: process_speak(text) def save(text): for t in text: save_("myfile", t) filecount = 0 def examples(): global text guide = f""" {text=} Examples: speak("A simple text") speak(text) # where text is a multiline string converted into list with splitlines() or a list of strings Example of saving the text into a file save_file("onefile", text)""".splitlines() for x in guide: print(x) pygame.init() pygame.mixer.init() # Provide a long text, making it a list text = """I've always thought too much it's like my brain cannot stop thinking so I guess what could stop overthinking """.splitlines() speak("This is working") speak(text) examples()
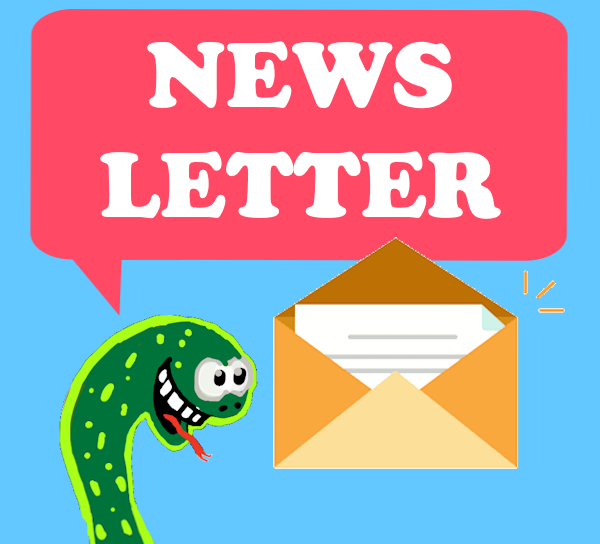


Twitter: @pythonprogrammi - python_pygame
Videos
Speech recognition gamePygame's Platform Game
