In this new post about tkinter, the module to create a GUI for python programming language: let’s see how to use the following three widgets with the tkinter module:
- Entry widget
- Label widget
- Listbox widget
An example, to start
In the following code we can see a little GUI to insert text in an entry, copy it into a label and a listbox at the same time.
Get the text in the entry widget
The entry widget is a texbox that can be used to get the input of the user. We need to get the vale that the user enters in the entry widget then. To get the text in the entry widget you need to follow this scheme:
- create a StringVar() object
- textvar = tkinter.Stringvar()
- link it to the entry with the argument
- e = Entry(root, textvariable=textvar)
- when the user hit return the function pass_value is called:
- entry.bind(“<Return>”, lambda x: pass_value())
The pass_value function gets the value of the texvar istance of StringVar with the method get:
- label[“text”] = textvar.get()
- listbox.insert(“1.0”, textvar.get())
So, this is the scheme to remember:
Scheme | ||
global scope | entry argument | how to get it |
v = tkinter.StringVar | textvariable=v | v.get() |
What is StringVar
Tkinter uses Tcl to use the widgets like labels, buttons etc.
To trace some values like the text in an entry widget, you can use a Tkinter variable with its constructor in this way:
var = StringVar()
It takes no value argument. To give it to it you can call the set method:
var.set(“hello”)
You can use this code to track the changes in the content of the widget that uses this var
def callback(*args): import tkinter as tk def callback(*args): print("Value changed") root = tk.Tk() var = tk.StringVar() var.trace("w", callback) entry = tk.Entry(root, textvariable=var) entry.pack() root.mainloop()
Let’s see the output of the code in this video.
Updating the value with callback function and trace method
We can use the trace method to do something useful like updating the value passed to a label, for example:
import tkinter as tk def callback(*args): print("Value changed") label['text'] = entry.get() root = tk.Tk() var = tk.StringVar() var.trace("w", callback) entry = tk.Entry(root, textvariable=var) entry.pack() label = tk.Label(root) label.pack() root.mainloop()
The example
In the following example we build a simple GUI with some widgets among wich we have the entry object with the StringVar() class taking trace of the value of the entry.
"""GUI example with entry A window with chance to put text into a label and a listbox """ # IMPORTING THE MODULE TKINTER import tkinter # THE WINDOW INSTANCE root = tkinter.Tk() # WIDTH AND HEIGHT + X AND Y OF THE SCREEN root.geometry("400x400+400+400") def pass_value(): """Passes the value into the label and the listbox""" # TAKES THE TEXT IN THE ENTRY (that hase the textvariable = textvar) label['text'] = textvar.get() # AND INSERT THAT TEXT INTO THE LB listbox.insert(tkinter.END, textvar.get()) # Delete the entry text textvar.set("") textvar = tkinter.StringVar() def entry(): """Creates an entry, a label and a listbox""" entry = tkinter.Entry(root, textvariable=textvar) entry['font'] = "Arial 28" entry.focus() entry.pack() entry.bind("<Return>", lambda x: pass_value()) label = tkinter.Label(root) label.pack() listbox = tkinter.Listbox(root) listbox.pack() return entry, label, listbox entry, label, listbox = entry() root.mainloop()
Get what the user inputs
We’ve seen how to insert a label and a button in a window with tkinter, the easiest module to make a GUI (graphic user interface) in Python. What now?
The entry widget
If we want the user to enter some text or numbers, we can use the Entry widget. The sintax is very similar to the one used for labels and buttons, but there is a great difference for the textvariable argument, that let’s us choose a variable in wich to store the text that the use input in the Entry widget. So, with the get() method we can grab this value and use it to elaborate it. But, shall we see some live coding in action?
Live coding in action: how to make and use an Entry widget in tkinter
Here is a little, but meaningful example, of how to put an Entry widget on the window and use a button to get and show (on a label) the content the user has entered into it. Let’s see how easy is that.
The code seen in this video
import tkinter root = tkinter.Tk() root.geometry("400x400+400+400") def label_value(): listbox.insert(tkinter.END, textvar.get()) entry.set("") textvar = tkinter.StringVar() entry = tkinter.Entry(root, textvariable=textvar) entry['font'] = "Arial 20" entry.focus() entry.pack() entry.bind("<Return>", lambda x: label_value()) listbox = tkinter.Listbox(root) listbox.pack() root.mainloop()
Commented code
import tkinter # initiate the window root = tkinter.Tk() # give width height top and left position root.geometry("400x400+400+400") def insert_value_in_list(): "insert as last item what inside entry (called when press enter)" listbox.insert(tkinter.END, textvar.get()) # and then clear the entry input box entry.set("") # this variable will get the text in the entry textvar = tkinter.StringVar() entry = tkinter.Entry(root, textvariable=textvar) # the character famuly and size entry['font'] = "Arial 20" # when starts it focus on the entry to input text immediately entry.focus() # make the entry visible entry.pack() # attach to the entry the event 'enter' to go to label_value function entry.bind("<Return>", lambda x: insert_value_in_list()) # add the listbox listbox = tkinter.Listbox(root) listbox.pack() root.mainloop()
Tkinter test for students
Tkinter articles
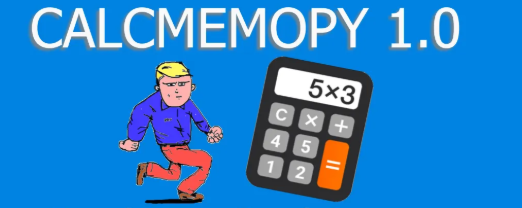