This app made in python with tkinter makes you read the latest news. You have to get the api key from https://newsapi.org/ making an account and saving a file called api_key.py with
apy_key = <your api key> # substitute with your api key
And then this script will make the work
from tkinter import * from tkinter import messagebox import requests import json from api_key import api_key type = 'sports' apiKey = api_key # BASE_URL = f'http://newsapi.org/v2/top-headlines?country={country,get()}&category={type}&apiKey='+apiKey class NewsApp: global apiKey, type def __init__(self, root): self.root = root self.root.geometry('1350x700+0+0') self.root.title("eNewsPaper") #====variables========# self.newsCatButton = [] self.newsCat = ["genral", "entertainment", "business", "sports", "technology", "health"] #========title frame===========# bg_color = "#404040" text_area_bg = "#b8e0c4" basic_font_color = "#ccc4c4" title = Label(self.root, text="NewsPaper Software", font=("times new roman", 30, "bold"), pady=2, bd=12, relief=GROOVE, bg=bg_color, fg=basic_font_color).pack(fill=X) F1 = LabelFrame(self.root, text="Category", font=( "times new roman", 20, "bold"), bg=bg_color, fg=basic_font_color, bd=10, relief=GROOVE) F1.place(x=0, y=80, width=300, relheight=0.88) for i in range(len(self.newsCat)): b = Button(F1, text=self.newsCat[i].upper( ), width=20, bd=7, font="arial 15 bold") b.grid(row=i, column=0, padx=10, pady=5) b.bind('<Button-1>', self.Newsarea) self.newsCatButton.append(b) #=======news frame=======# F2 = Frame(self.root, bd=7, relief=GROOVE) F2.place(x=320, y=80, relwidth=0.7, relheight=0.8) Label(F2, text="Country").pack() self.v = StringVar() self.country = Entry(F2, textvariable=self.v) self.v.set("it") self.country.pack() news_title = Label(F2, text="News Area", font=( "arial", 20, "bold"), bd=7, relief=GROOVE).pack(fill=X) scroll_y = Scrollbar(F2, orient=VERTICAL) self.txtarea = Text(F2, yscrollcommand=scroll_y.set, font=( "times new roman", 15, "bold"), bg=text_area_bg, fg="#3206b8") scroll_y.pack(side=RIGHT, fill=Y) scroll_y.config(command=self.txtarea.yview) self.txtarea.insert( END, "PLEASE SELECT ANY CATEGORY TO SHOW HEADLINES AND PLEASE BE PATIENT IT DEPENDS ON UR INTERNET CONNECTIONS!!!!") self.txtarea.pack(fill=BOTH, expand=1) def Newsarea(self, event): type = event.widget.cget('text').lower() BASE_URL = f'http://newsapi.org/v2/top-headlines?country={self.country.get()}&category={type}&apiKey='+apiKey self.txtarea.delete("1.0", END) self.txtarea.insert(END, f"\n Welcome to GFG news paper\n") self.txtarea.insert( END, "--------------------------------------------------------------------\n") try: articles = (requests.get(BASE_URL).json())['articles'] if(articles != 0): for i in range(len(articles)): self.txtarea.insert(END, f"{articles[i]['title']}\n") self.txtarea.insert( END, f"{articles[i]['description']}\n\n") self.txtarea.insert(END, f"{articles[i]['content']}\n\n") self.txtarea.insert( END, f"read more...{articles[i]['url']}\n") self.txtarea.insert( END, "--------------------------------------------------------------------\n") self.txtarea.insert( END, "--------------------------------------------------------------------\n") else: self.txtarea.insert(END, "Sorry no news available") except Exception as e: messagebox.showerror( 'ERROR', "Sorry cant connect to internet or some issues with newsapp :'(") root = Tk() obj = NewsApp(root) root.mainloop()
The codes for the country, to set the news from one specific country
Afghanistan AF Akrotiri and Dhekelia – See United Kingdom, The Åland Islands AX Albania AL Algeria DZ American Samoa AS Andorra AD Angola AO Anguilla AI Antarctica [a] AQ Antigua and Barbuda AG Argentina AR Armenia AM Aruba AW Ashmore and Cartier Islands – See Australia. Australia [b] AU Austria AT Azerbaijan AZ Bahamas (the) BS Bahrain BH Bangladesh BD Barbados BB Belarus BY Belgium BE Belize BZ Benin BJ Bermuda BM Bhutan BT Bolivia (Plurinational State of) BO Bonaire BQ Sint Eustatius Saba Bosnia and Herzegovina BA Botswana BW Bouvet Island BV Brazil BR British Indian Ocean Territory (the) IO British Virgin Islands – See Virgin Islands (British). Brunei Darussalam [e] BN Bulgaria BG Burkina Faso BF Burma – See Myanmar. Burundi BI Cabo Verde [f] CV Cambodia KH Cameroon CM Canada CA Cape Verde – See Cabo Verde. Caribbean Netherlands – See Bonaire, Sint Eustatius and Saba. Cayman Islands (the) KY Central African Republic (the) CF Chad TD Chile CL China CN China, The Republic of – See Taiwan (Province of China). Christmas Island CX Clipperton Island – See France. Cocos (Keeling) Islands (the) CC Colombia CO Comoros (the) KM Congo (the Democratic Republic of the) CD Congo (the) [g] CG Cook Islands (the) CK Coral Sea Islands – See Australia. Costa Rica CR Côte d'Ivoire [h] CI Croatia HR Cuba CU Curaçao CW Cyprus CY Czechia [i] CZ Democratic People's Republic of Korea – See Korea, The Democratic People's Republic of. Democratic Republic of the Congo – See Congo, The Democratic Republic of the. Denmark DK Djibouti DJ Dominica DM Dominican Republic (the) DO East Timor – See Timor-Leste. Ecuador EC Egypt EG El Salvador SV England – See United Kingdom, The. Equatorial Guinea GQ Eritrea ER Estonia EE Eswatini [j] SZ Ethiopia ET Falkland Islands (the) [Malvinas] [k] FK Faroe Islands (the) FO Fiji FJ Finland FI France [l] FR French Guiana GF French Polynesia PF French Southern Territories (the) [m] TF Gabon GA Gambia (the) GM Georgia GE Germany DE Ghana GH Gibraltar GI Great Britain – See United Kingdom, The. Greece GR Greenland GL Grenada GD Guadeloupe GP Guam GU Guatemala GT Guernsey GG Guinea GN Guinea-Bissau GW Guyana GY Haiti HT Hawaiian Islands – See United States of America, The. Heard Island and McDonald Islands HM Holy See (the) [n] VA Honduras HN Hong Kong HK Hungary HU Iceland IS India IN Indonesia ID Iran (Islamic Republic of) IR Iraq IQ Ireland IE Isle of Man IM Israel IL Italy IT Ivory Coast – See Côte d'Ivoire. Jamaica JM Jan Mayen – See Svalbard and Jan Mayen. Japan JP Jersey JE Jordan JO Kazakhstan KZ Kenya KE Kiribati KI Korea (the Democratic People's Republic of) [o] KP Korea (the Republic of) [p] KR Kuwait KW Kyrgyzstan KG Lao People's Democratic Republic (the) [q] LA Latvia LV Lebanon LB Lesotho LS Liberia LR Libya LY Liechtenstein LI Lithuania LT Luxembourg LU Macao [r] MO North Macedonia [s] MK Madagascar MG Malawi MW Malaysia MY Maldives MV Mali ML Malta MT Marshall Islands (the) MH Martinique MQ Mauritania MR Mauritius MU Mayotte YT Mexico MX Micronesia (Federated States of) FM Moldova (the Republic of) MD Monaco MC Mongolia MN Montenegro ME Montserrat MS Morocco MA Mozambique MZ Myanmar [t] MM Namibia NA Nauru NR Nepal NP Netherlands (the) NL New Caledonia NC New Zealand NZ Nicaragua NI Niger (the) NE Nigeria NG Niue NU Norfolk Island NF North Korea – See Korea, The Democratic People's Republic of. Northern Ireland – See United Kingdom, The. Northern Mariana Islands (the) MP Norway NO Oman OM Pakistan PK Palau PW Palestine, State of PS Panama PA Papua New Guinea PG Paraguay PY People's Republic of China – See China. Peru PE Philippines (the) PH Pitcairn [u] PN Poland PL Portugal PT Puerto Rico PR Qatar QA Republic of China – See Taiwan (Province of China). Republic of Korea – See Korea, The Republic of. Republic of the Congo – See Congo, The. Réunion RE Romania RO Russian Federation (the) [v] RU Rwanda RW Saba – See Bonaire, Sint Eustatius and Saba. Sahrawi Arab Democratic Republic – See Western Sahara. Saint Barthélemy BL Saint Helena SH Ascension Island Tristan da Cunha Saint Kitts and Nevis KN Saint Lucia LC Saint Martin (French part) MF Saint Pierre and Miquelon PM Saint Vincent and the Grenadines VC Samoa WS San Marino SM Sao Tome and Principe ST Saudi Arabia SA Scotland – See United Kingdom, The. Senegal SN Serbia RS Seychelles SC Sierra Leone SL Singapore SG Sint Eustatius – See Bonaire, Sint Eustatius and Saba. Sint Maarten (Dutch part) SX Slovakia SK Slovenia SI Solomon Islands SB Somalia SO South Africa ZA South Georgia and the South Sandwich Islands GS South Korea – See Korea, The Republic of. South Sudan SS Spain ES Sri Lanka LK Sudan (the) SD Suriname SR Svalbard SJ Jan Mayen Sweden SE Switzerland CH Syrian Arab Republic (the) [x] SY Taiwan (Province of China) [y] TW Tajikistan TJ Tanzania, the United Republic of TZ Thailand TH Timor-Leste [aa] TL Togo TG Tokelau TK Tonga TO Trinidad and Tobago TT Tunisia TN Turkey TR Turkmenistan TM Turks and Caicos Islands (the) TC Tuvalu TV Uganda UG Ukraine UA United Arab Emirates (the) AE United Kingdom of Great Britain and Northern Ireland (the) GB United States Minor Outlying Islands (the) [ac] UM United States of America (the) US United States Virgin Islands – See Virgin Islands (U.S.). Uruguay UY Uzbekistan UZ Vanuatu VU Vatican City – See Holy See, The. Venezuela (Bolivarian Republic of) VE Viet Nam [ae] VN Virgin Islands (British) [af] VG Virgin Islands (U.S.) [ag] VI Wales – See United Kingdom, The. Wallis and Futuna WF Western Sahara [ah] EH Yemen YE Zambia ZM Zimbabwe ZW
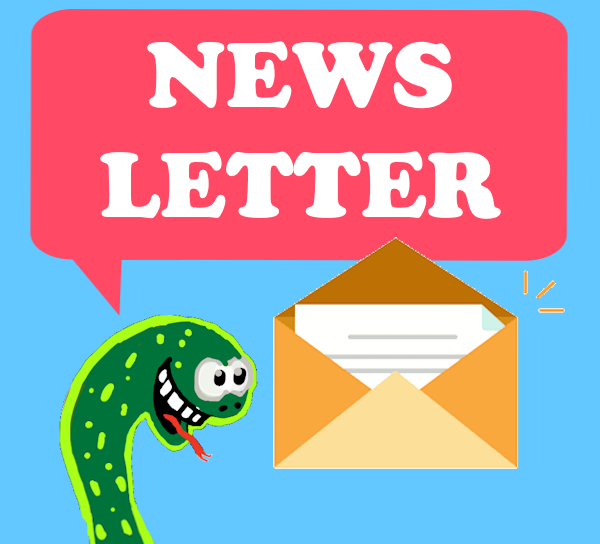


Twitter: @pythonprogrammi - python_pygame
Videos
Speech recognition gamePygame's Platform Game
