Hi everybody, today we want to use Python and tkinter to evaluate a students test in a fast and easy way. You will see the details in the following video, but I want to tell you that this is the first part of a little project, so you will see other posts about this topic soon.
What is this about?
We are talking about tests for students in which we use an evaluation grid to give it a grade based on some paramenters: knowledge, elaboration and expressiveness (for example). Each one of this parameters (or indicators) can have a evaluation in a range of 5, 3 and 2 respectively.
Tkinter
Tkinter is the built in module of Python to make GUI, graphical user interface. This video is a good example about making a little less basic use of the widget. For a more basic use of tkinter go search into this blog, you will find a lot of practical example (go also in the links at the bottom of this page).
import tkinter as tk root = tk.Tk() def name_it(frame_root): "Label and entry with the name" frame1 = frame_root lab_name = tk.Label(frame1, text="Name:") lab_name.pack(side="left") name = tk.Entry(frame1) name.pack() return frame1 v = [] counter = -1 def entry(frame1): global counter counter += 1 v.append(tk.IntVar()) return tk.Entry(frame1, textvariable=v[counter]).pack(side="left") def question(frame_root, num): "Questions frame" frame1 = frame_root lab_name = tk.Label(frame1, text="Question {}:".format(num)) lab_name.pack(side="left") for n in range(3): entry(frame1) return frame1 def calculate(): values = [n.get() for n in v] sumval = sum(values) print(sumval) print("In a scale of 10: ", sumval / 4) frame1 = name_it(tk.Frame(root)) frame1.pack() for n in range(4): question(tk.Frame(root), n + 1).pack() button = tk.Button(root, text="Calculate points", command=calculate) button.pack() root.mainloop()
Other posts about tkinter
I talked about tkinter in these posts:
Tkinter test for students
Tkinter articles
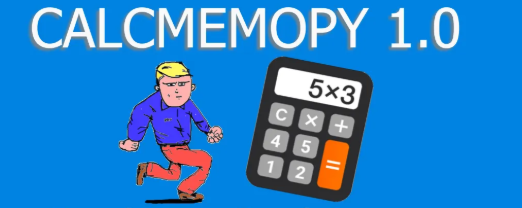