Let’s start making a nice and clean window with tkinter to make an app that creates different tests for every students. The test will use a template and have different, random, data for every student. At the end it will create a file for the students and a file with the solution for the teacher. This is the first part. We will try to make a nice class for the GUI, so that we can reuse most of the code to speed up the work and make it easy to adjust for out purposes that may change as we go further and we also want to make it suitable for different type of test and for different type of classrooms and students.
This is the first part of the code with the video of the live coding in the section below. This could be also a very nice example to see how tkinter works with entry and label widgets, with the grid system and how you can manage the widget in a more object oriented style, using a class, rather than writing code in other messy ways, because, with all the parameters you got to take care of for the widgets, it can became very messy to have a lot of them in a window. So, let’s start.
import tkinter as tk class Window: def __init__(self): self.root = tk.Tk() self.mem = {} def label(self, text, r, c): "Creates a label with a text and row and column as arguments" self.label = tk.Label( self.root, text=text) self.label.grid(row=r, column=c) return self.label def entry(self, r, c): "Creates an input entry for the user" self.v = tk.StringVar() self.e = tk.Entry( self.root, textvariable=self.v) self.e.grid(row=r, column=c) return self.e def memorize(self, data): self.mem[data] = self.v.get() print(self.mem) def number_of_students(): "Creates the input of number of students" label = w.label("Number of stundents", 0, 0) entry = w.entry(0, 1) entry.bind("<Return>", lambda x: w.memorize(label["text"])) w = Window() number_of_students() w.root.mainloop()
As you can see, the code is not too much and we will have not much code even when we are going to add more widgets in it, so that I can add features in the future at ease.
The video with live coding
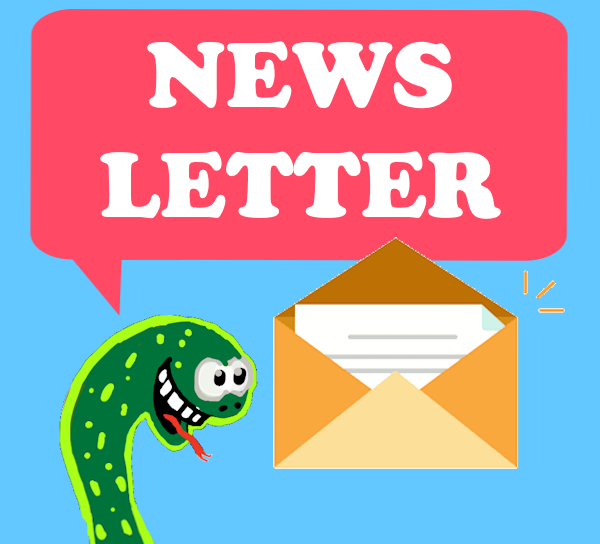


Twitter: @pythonprogrammi - python_pygame
Videos
Speech recognition gamePygame's Platform Game
