In this version of the mini-calculator seen in the latest post we added a twist… the reminder of the operations made in a listbox. Next post we will add the chance to save the list of operations… how cool is this?
import tkinter as tk # This class inherit from Frame class App(tk.Frame): def __init__(self): tk.Frame.__init__(self) self.option_add("*Font", "arial 20 bold") self.pack(expand=tk.YES, fill=tk.BOTH) self.master.title("Calculator") # the widgets: display, clear button... self._display() self.list_of_ops() def _display(self): display = tk.StringVar() entry = tk.Entry( self, relief=tk.FLAT, textvariable=display, justify='right', bd=15, bg='orange') entry.pack(side=tk.TOP) entry.focus() self.master.bind("<Return>", lambda x: self.calc(display)) self.master.bind("<Escape>", lambda x: display.set("")) def list_of_ops(self): self.listbox = tk.Listbox(self) self.listbox.pack() def calc(self, display): try: ris = eval(display.get()) if str(display.get()) == str(ris): display.set("") else: self.listbox.insert(tk.END, display.get() + "=" + str(ris)) display.set(ris) except NameError as e: display.set("ERR: press ESC") if __name__ == '__main__': App().mainloop()
Put an icon in the window
If you want to put and icon (a png in this case) you can add this code to the code above, in the def __init__ method, after giving the title to the window:
self.master.title("Calculator") self.master.tk.call('wm', 'iconphoto', self.master._w, tk.PhotoImage(file='calculator.png'))
The result will be this, putting this image, for example, in the same folder of the python script
In the next episode:
– dark mode
– delete items form the list
– save a list of operations with a name in a text file
– memorize some results in a list to sum them later
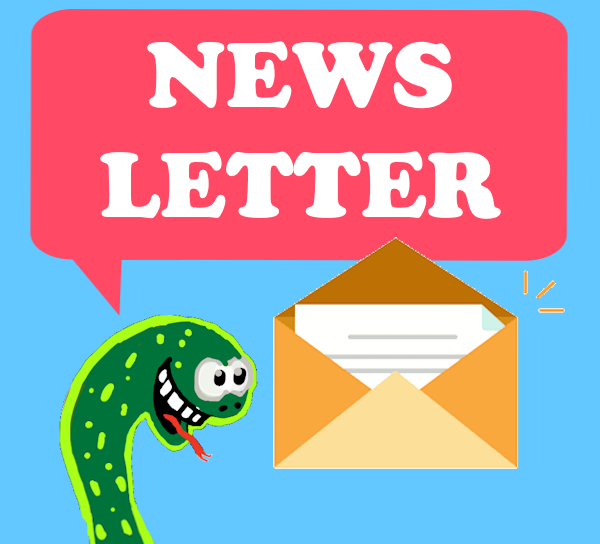


Twitter: @pythonprogrammi - python_pygame
Videos
Speech recognition gamePygame's Platform Game
