Bind a key (any key)
import tkinter as tk keyspressed = 0 def key(): global keyspressed print(f"Pressed a key: {keyspressed} times") keyspressed += 1 root = tk.Tk() root.bind("<Key>", lambda x: key()) label = tk.Label(root, text="Press a key and look at the console") label.pack() root.mainloop()
Press a particular key
root.bind("<c>", lambda x: key())
Press ctrl + a key
root.bind("<Control-c>", lambda x: key())
Select an item in the listbox
def sel(): print("An item has been selected") listbox = tk.Listbox(root) listbox.pack() listbox.bind("<<ListboxSelect>>", lambda x: sel())
Double click on an item in a listbox
def double_clicked(): print("An item has been double clicked") listbox = tk.Listbox(root) listbox.pack() listbox.bind("<Double-Button>", lambda x: double_clicked())
Key binding with tkinter
In another post we’ve yet seen how to bind the click of a mouse(<Button-1>) to intercept the user action and make the computer do something. We also tryied to apply the code to an example of a little game.
Now we want to see another example, but with the use of Key (<Key>) binding.
A basic example of Key binding
This example show you how is the syntax to bind a key event and how we can grab the key pressed showing it to the console. In the next video we will add some code to make a more complex example of its possible use.
Video number 2
In this video we will see the char pressed in a label.
Video nr. 3
In the following video, pressing “a” the width of the frame will shrink.
Tkinter test for students
Tkinter articles
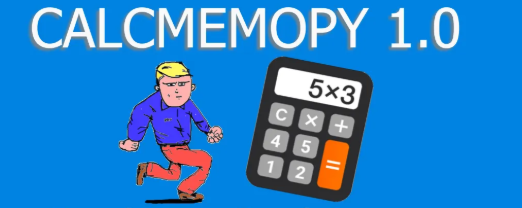