Can I add an image to the window?
An image is better than ten thousand words. This being said, let’s see how to see an image with Python in the shortest way. You may want to use this image in an application. That is why we are going to use the built-in module tkinter. Buil-in means that you got it from the start of your fresh installation of Python, you do not have to install nothing but Python to have it. The only thing you need to do is to import it. You can import tkinter (Tkinter for python 2) in many way.
from tkinter import *
From tkinter import * (aka everything) is the easiest way, but not the better. In this way you can use the tkinter widgets without having to put tkinter.Label(… but just Label(… to create a label. It is not very convenient to use * because all the functions and classes of the module tkinter being accessible without anything before can overwrite other functions or classes with the same name in Python or in other imported modules. For little projects or scripts this may not sound a big deal, but for more complex project it could be very difficult to find bugs having imported many modules with th * thing. So… do not use it apart from fast experiments within the interpreter, saving some characters does not worth the risk.
So… instead of this:
from tkinter import * root = Tk() # ============================== the code ============== # 1. CREATE AN IMAGE OBJECT photoImageObj = PhotoImage(file="code_girl01.png") # 2. THEN YOU CREATE A LABEL WITH image = photoImageObj lab = Label(root, image=photoImageObj).pack() # ==================================================pp== root.mainloop()
we will make this
import tkinter as tk root = tk.Tk() # ============================== the code ============== # 1. CREATE AN IMAGE OBJECT photoImageObj = tk.PhotoImage(file="code_girl01.png") # 2. THEN YOU CREATE A LABEL WITH image = photoImageObj lab = tk.Label(root, image=photoImageObj).pack() # ==================================================pp== root.mainloop()
Another advantage is that when you read the code you know exactly where that function or class belongs.
This is the image used in the code above.
This is the output window of the code above
Let’s go beyond this simple code snippet
As usual, we will now try to see how we can take advantage of the simple code we start from to make something more complex and useful. Could we make a photoviewer using this starting point? What we should do? I am gonna make a point:
- look in the dir (glob module) and create a list of the images
- create an object PhotoImage of the first image in the list of files
- charge it in the label (it’s the code we’ve seen above)
- bind the click of a mouse to change the image in the label
- new object PhotoImage
Let’s do it.
What is glob
This module let me get a list of the files that are in a folder and can let me easily choose some kind of file with this code, for example,:
import glob list_of_png = glob.glob("*.png")
Here it is again the * symbol that means everything or any. In this case it says: get me any file that starts with every name (so any name) and ends with a .png extention, so that it will return me a list (memorized in the variable list_of_png) with all the names of the png files in the folders. I can use this method for any kind of file, but this time I want png files (that are images). Rember not to call a variable just list, because list is a built-in function and if you use it as a variable name you will overwrite the built-in function not having the chance to use it in your script (but do not worry it is not a permanent thing in any case, when you will restart python the function list will be there for you).
An image browser
This time we create an app to browse images with tkinter. This code will be explained in a future post.
import tkinter as tk import glob from PIL import ImageTk root = tk.Tk() # ============================== the code ============== list_png = glob.glob("*.png") photo = ImageTk.PhotoImage(file="code_girl01.png") # 2. THEN YOU CREATE A LABEL WITH image = photoImageObj lab = tk.Label(root, image=photo) lab.image = photo lab.pack() name = tk.StringVar() lb = tk.Listbox(root) for img in list_png: lb.insert(0,img) lb.pack() x = 0 def go(event): global x lb.itemconfig(x, bg="") x += 1 photo = ImageTk.PhotoImage(file = list_png[x]) lab['image'] = photo lab.image = photo # ====== !!! THIS IS IMPORTANT TO SEE THE IMAGES !!! ===== lb.curselection = list_png[x] print(lb.curselection) lb["selectbackground"] = "yellow" lb.itemconfig(x, bg="yellow") #lb.bind("<Double-Button>", lambda event: change(event)) lab.bind("<Button-1>", lambda event: go(event)) # ==================================================pp== root.mainloop()
Tkinter test for students
Tkinter articles
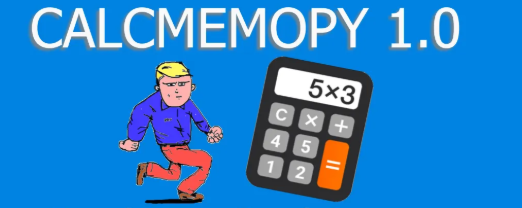