Below is an example of creating a simple window using Tkinter with an Entry widget, a Text widget, and a Button widget. You’ll need to have Python installed to run this code, tkinter comes with python:
import tkinter as tk def on_button_click(): text = entry.get() text_widget.insert(tk.END, f"Text entered: {text}\n") entry.delete(0, tk.END) # Create the main window window = tk.Tk() window.title("Tkinter Window") # Entry widget entry = tk.Entry(window, width=30) entry.pack(pady=10) # Text widget text_widget = tk.Text(window, width=30, height=10) text_widget.pack() # Button widget button = tk.Button(window, text="Submit", command=on_button_click) button.pack(pady=10) # Start the main event loop window.mainloop()
Save this code in a file with a .py
extension (e.g., tkinter_example.py
), and then run it using Python. A window will open with an Entry widget, a Text widget, and a Button widget. When you type something in the Entry widget and click the button, the text will be displayed in the Text widget, and the Entry widget will be cleared.
Please note that Tkinter is a standard Python library for creating GUI applications. It might not have the most modern look and feel, but it’s straightforward to use for simple applications like this one. For more sophisticated GUIs, you may want to explore other libraries like PyQt or wxPython.
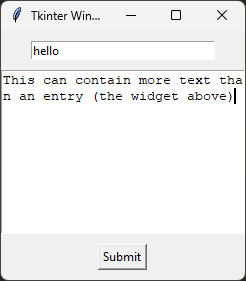
A version with a class
import tkinter as tk # Create the main window class Window: def __init__(self): self.window = tk.Tk() self.window.title("Tkinter Window") # Entry widget self.entry = tk.Entry(self.window, width=30) self.entry.pack(pady=10) # Text widget self.text_widget = tk.Text(self.window, width=30, height=10) self.text_widget.pack() # Button widget self.button = tk.Button(self.window, text="Submit", command=self.on_button_click) self.button.pack(pady=10) self.window.mainloop() def on_button_click(self): self.text = self.entry.get() self.text_widget.insert(tk.END, f"Text entered: {self.text}\n") self.entry.delete(0, tk.END) win = Window()
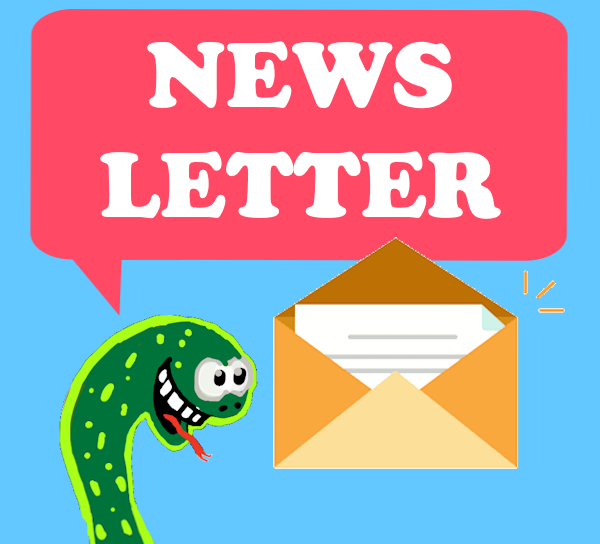


Twitter: @pythonprogrammi - python_pygame
Videos
Speech recognition gamePygame's Platform Game
