
This Python code creates a simple graphical user interface (GUI) using the tkinter library. The GUI consists of a window with a yellow background and a blue text color, a large text area (textarea), and a button that says “Delete text.” The purpose of the GUI is to allow users to enter and delete text.
The code is organized into several functions. The textarea
function creates the text area, sets its color and font, and binds the delete_action
function to the Ctrl+x
keyboard shortcut. The delete_button
function creates the button and sets its font and command to call the delete_action
function.
The window
function combines these functions to create the GUI window, sets the window title to “My notebook – ctrl+x to delete,” and starts the event loop with the mainloop()
method.
The delete_action
function is called when the “Delete text” button is pressed or the Ctrl+x
keyboard shortcut is used. It simply deletes all the text in the textarea by calling the delete()
method with the arguments "0.0"
(beginning of the text) and tk.END
(end of the text).
Overall, this code demonstrates how to create a simple GUI with tkinter, including a text area, a button, and keyboard shortcuts, and how to handle user events such as button clicks and key presses.
import tkinter as tk def window(): ''' A window with a textarea and a button to delete ''' def delete_action(event=0): ''' function to delete text called pressing delete_button (but)''' ta.delete("0.0", tk.END) def textarea(): ''' text widget ''' ta = tk.Text( root, bg="yellow", fg="blue", font="Arial 20" ) ta.pack() ta.focus() ta.bind("<Control-x>", delete_action) return ta def delete_button(): ''' button to trigger the delete action ''' but = tk.Button( root, text="Delete text", font="Arial 20", command=delete_action) but.pack() # loop that starts the window root = tk.Tk() root.title("My notebook - ctrl+x to delete") ta = textarea() # create text widget delete_button() root.mainloop() if __name__
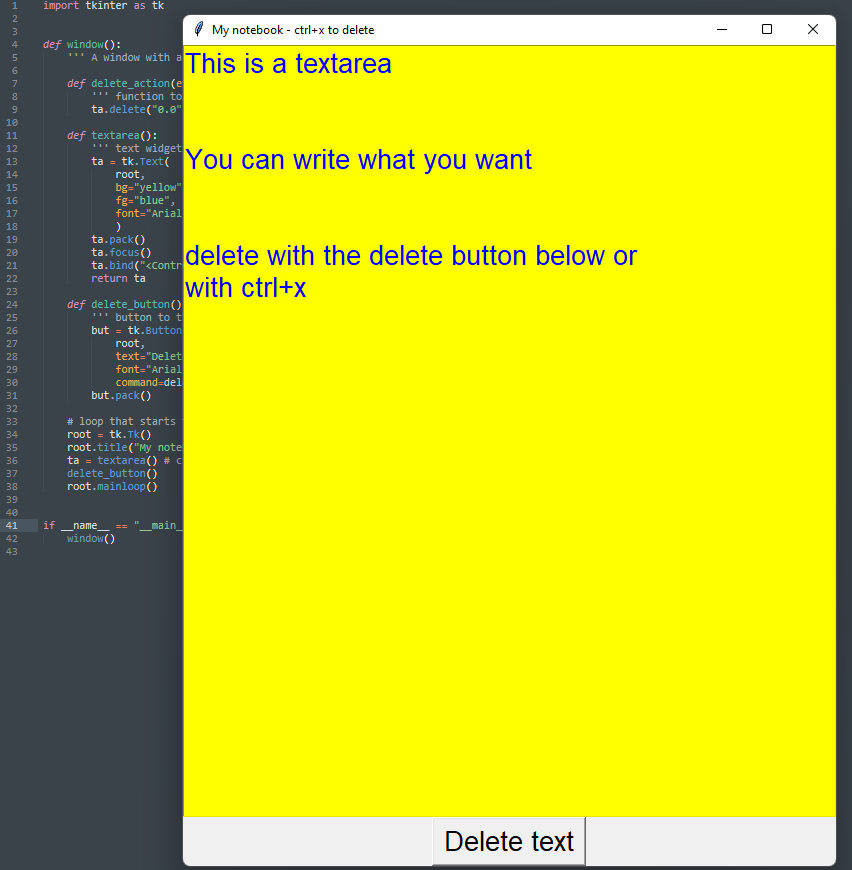
A window with a button
This code is an example of a simple GUI (graphical user interface) application built using the Tkinter module in Python. Tkinter is a standard Python library used for building desktop applications with graphical interfaces.
The code starts with importing the Tkinter module as tk
. Next, a function named hello()
is defined, which is called whenever the button is clicked. The function changes the text of the button to “You clicked” when the button is clicked.
After that, an instance of the Tk
class is created using root = tk.Tk()
. The Tk
class is the top-level widget of the Tkinter GUI toolkit, which represents the main window of the application.
A button widget is created using the Button
class, with the parent widget as root
, text as “Click”, and the command to execute as hello
. The command
parameter is used to specify the function to be called when the button is clicked.
Finally, the button widget is packed using the pack()
method, which arranges the widget within the parent widget.
The mainloop()
method is called to start the main event loop of the application, which waits for user input and responds to it accordingly.
Overall, this code is a simple example of how to create a basic GUI application using Tkinter in Python. With some modifications and additional widgets, you can create more complex and feature-rich GUI applications.
import tkinter as tk def hello(): but["text"] = "You clicked" root = tk.Tk() but = tk.Button( root, text="Click", command=hello) but.pack() root.mainloop()


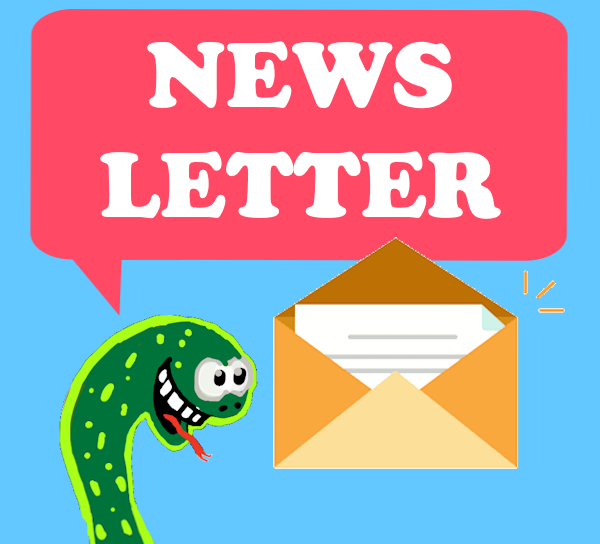


Twitter: @pythonprogrammi - python_pygame
Videos
Speech recognition gamePygame's Platform Game
