Let’s see how to display this map on the screen with pygame.
We need 2 images (you can get them in the repository with the code: https://github.com/formazione/platformworlds in the folder “display map only”
https://github.com/formazione/platformworlds/tree/master/display%20map%20only


This map is made with this string
map = """ oo xxxxxxx xx xxxxx o o o oo ooooo oo oo o o o oo ooo oo oo ooooooo o oo o o oo oo ooo ooooo oo o o o oo oooooooooooooooooooooooo oooooooooooooooooooooooo oooooooooooooooooooooooo """.splitlines()
The x represent the grass image and the o represent the dirt image.
The images are loaded here
tl = {} tl["o"] = pygame.image.load('dirt.png') tl["x"] = pygame.image.load('grass.png')
In this dictionary, where the name of the images x and o are the keys. This is good if you got few images for the tiles, but if you want hundreds of tiles you should find a different way to make maps, rather that using letters in a string. We will solve this problem making a map editor very soon.
Ok the code is in the repository that I showed above, and it’s this:
import pygame import sys from pygame.locals import * map = """ oo xxxxxxx xx xxxxx o o o oo ooooo oo oo o o o oo ooo oo oo ooooooo o oo o o oo oo ooo ooooo oo o o o oo oooooooooooooooooooooooo oooooooooooooooooooooooo oooooooooooooooooooooooo """.splitlines() tl = {} tl["o"] = pygame.image.load('dirt.png') tl["x"] = pygame.image.load('grass.png') pygame.init() pygame.display.set_caption('Game') WINDOW_SIZE = (764,368) screen = pygame.display.set_mode(WINDOW_SIZE, 0, 32) display = pygame.Surface((WINDOW_SIZE[0] // 2, WINDOW_SIZE[1] // 2)) clock = pygame.time.Clock() loop = 1 while loop: # CLEAR THE SCREEN display.fill((146, 244, 255)) for event in pygame.event.get(): if event.type == QUIT: loop = 0 # Tiles are blitted ========================== tile_rects = [] y = 0 for line_of_symbols in map: x = 0 for symbol in line_of_symbols: if symbol != " ": display.blit(tl[symbol], (x * 16, y * 16)) # draw a rectangle for every symbol except for the empty one x += 1 y += 1 screen.blit(pygame.transform.scale(display, WINDOW_SIZE), (0, 0)) pygame.display.update() clock.tick(60) pygame.quit()
How to show the tiles
Coding is not about remembering the syntax of a language, but it’s about problem solving. One can be how to show the tiles.
In the code above I adopted this solution:
# Tiles are blitted ========================== tile_rects = [] y = 0 for line_of_symbols in map: x = 0 for symbol in line_of_symbols: if symbol != " ": display.blit(tl[symbol], (x * 16, y * 16)) # draw a rectangle for every symbol except for the empty one x += 1 y += 1
Previously, instead I used a different approach. We will see this one in the next post.
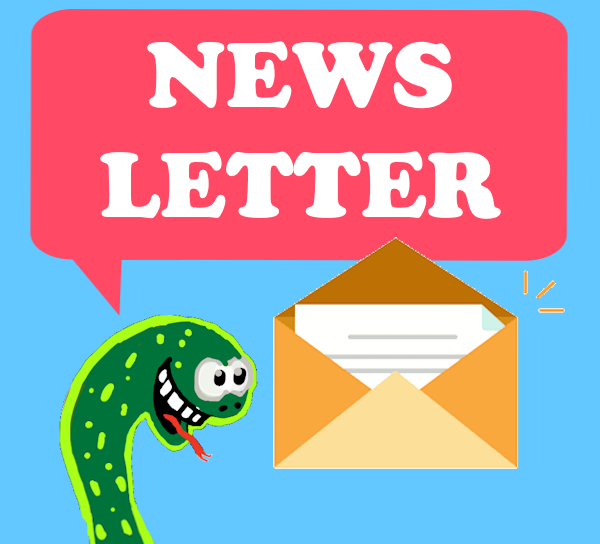


Twitter: @pythonprogrammi - python_pygame
Videos
Speech recognition gamePygame's Platform Game

Other Pygame's posts
