This is a very basic program for pygame module to show an image full screen in FULLSCREEN mode. The image will be stretched to the full screen and you will not be able to see the button to close the window, so we will draw a button (with a red rectangle sa background and 2 lines to make the X, not using fonts to save time and code, maybe). The “button” is easily resizable. To do so you just need to change the pygame.Rect coordinates and the lines coordinates will change accordingly to the dimension of the rectangle. When you click on the button the window closes itself, just like the native red button of the window. Here is the code
# full screen image with exit button drawed import pygame pygame.init() wh = width, height = 800,600#pygame.display.get_desktop_sizes()[0] print(width, height) screen = pygame.display.set_mode((wh), )#pygame.FULLSCREEN) def bg(): image = pygame.image.load("image.png") image_scaled = pygame.transform.scale(image, (wh)) screen.blit(image_scaled, (0, 0)) # Or use image if not scaled def exit_button(): exit_button_rect = pygame.Rect(10, 10, 50, 50) # Create a rectangle for the button exit_button_color = (255, 0, 0) # Red color for the button pygame.draw.rect(screen, exit_button_color, exit_button_rect) pygame.draw.line(screen, (255,255,255), (exit_button_rect[0],exit_button_rect[1]), (exit_button_rect[0]+exit_button_rect[2],exit_button_rect[1]+exit_button_rect[3]), 3 ) pygame.draw.line(screen, (255,255,255), (exit_button_rect[0]+exit_button_rect[2],exit_button_rect[1]), (exit_button_rect[0],exit_button_rect[1]+exit_button_rect[3]), 3 ) return exit_button_rect bg() exit_button_rect = exit_button() pygame.display.flip() running = True while running: for event in pygame.event.get(): if event.type == pygame.QUIT: running = False if event.type == pygame.MOUSEBUTTONDOWN: if exit_button_rect.collidepoint(event.pos): running = False pygame.quit()
You can find it in the following repository (the file is called full_image_exit.py):
https://github.com/formazione/pygame_tutorial.git
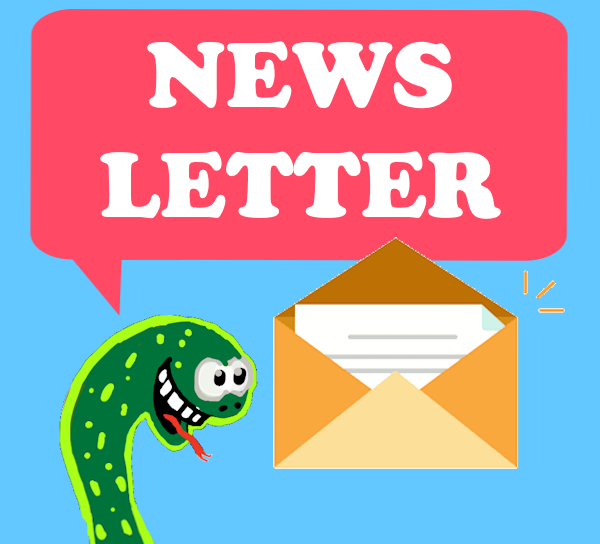


Twitter: @pythonprogrammi - python_pygame
Videos
Speech recognition gamePygame's Platform Game

Other Pygame's posts
