You may know that chat gpt can create code. So I asked to make some code to make multiple windows with tkinter, to see how he would have answered. This is the answer
Here is an example of how you could create multiple windows using tkinter, the built-in Python GUI framework:
import tkinter as tk class MainWindow(tk.Tk): def __init__(self): super().__init__() self.button = tk.Button(self, text="Create new window", command=self.create_window) self.button.pack() self.windows = [] def create_window(self): new_window = tk.Toplevel(self) self.windows.append(new_window) # You can now add widgets to the new window if __name__ == "__main__": window = MainWindow() window.mainloop()

In this example, pressing the button in the main window creates a new window using the Toplevel
widget. The new window will be added to a list of windows, so that you can keep track of all the windows that have been created. You can then add any widgets you want to the new window.
Note that in order to create a new window, you need to create a new instance of the Toplevel
class, and pass the parent window as the first argument to the constructor. This will make the new window a child of the parent window.
I hope this helps! Let me know if you have any other questions.
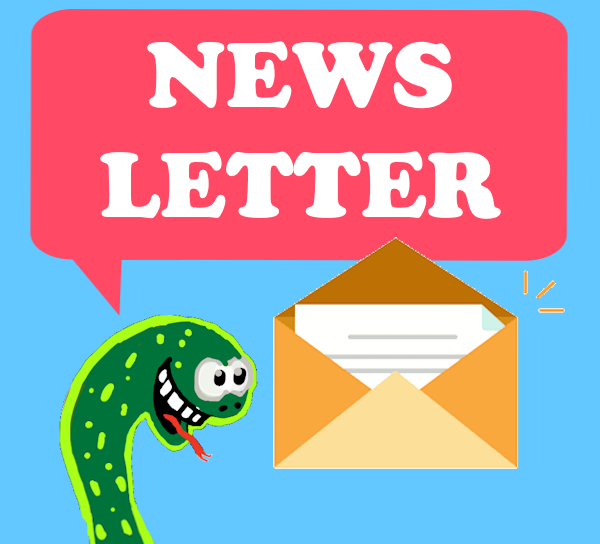


Twitter: @pythonprogrammi - python_pygame
Videos
Speech recognition gamePygame's Platform Game
