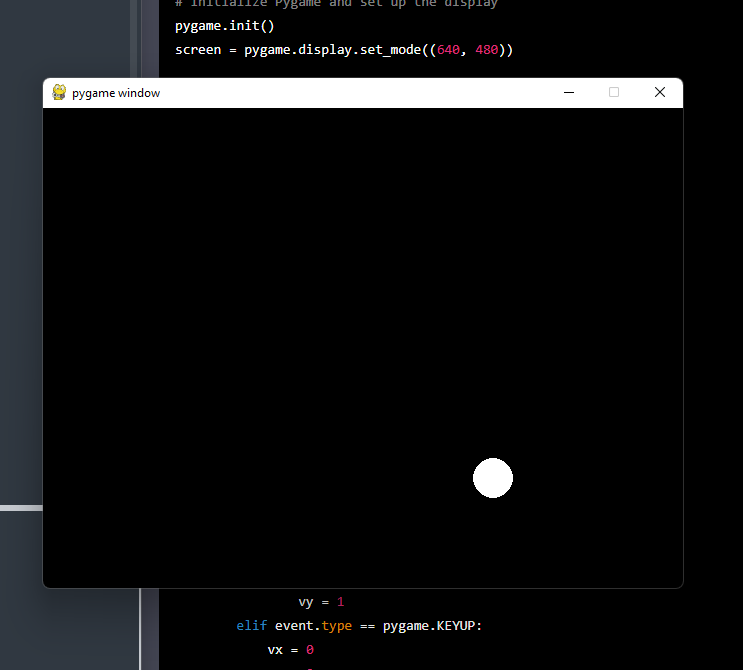
To create a ball that moves on the screen with Python, you will need to use a combination of the following tools:
- A graphics library, such as Pygame, to draw and update the display
- A loop to continuously update the ball’s position and redraw the screen
- Variables to store the ball’s position and velocity (movement in a given direction)
- Input handling to allow the user to control the ball’s movement
Here is an example of how you might go about creating a moving ball with Pygame:
import pygame # Initialize Pygame and set up the display pygame.init() screen = pygame.display.set_mode((640, 480)) clock = pygame.time.Clock() # Set up the ball's initial position and velocity x = 320 y = 240 vx = 1 vy = 1 # Run the game loop running = True while running: # Handle user input for event in pygame.event.get(): if event.type == pygame.QUIT: running = False elif event.type == pygame.KEYDOWN: if event.key == pygame.K_LEFT: vx = -1 elif event.key == pygame.K_RIGHT: vx = 1 elif event.key == pygame.K_UP: vy = -1 elif event.key == pygame.K_DOWN: vy = 1 elif event.type == pygame.KEYUP: vx = 0 vy = 0 # Update the ball's position x += vx y += vy # Draw the ball and update the display screen.fill((0, 0, 0)) pygame.draw.circle(screen, (255, 255, 255), (x, y), 20) pygame.display.flip() clock.tick(60) # Quit Pygame when the game loop is finished pygame.quit()
Ok.
This code creates a Pygame window with a white ball in the center. The ball moves in the direction specified by the vx
and vy
variables, which can be controlled by the arrow keys. When the arrow keys are released, the ball stops moving. The ball bounces off the edges of the window when it reaches them.
To prevent the ball from going off the screen, you can add some code to check the ball’s position and reverse its velocity if it goes out of bounds. For example:
import pygame # Initialize Pygame and set up the display pygame.init() screen = pygame.display.set_mode((640, 480)) clock = pygame.time.Clock() # Set up the ball's initial position and velocity x = 320 y = 240 vx = 1 vy = 1 # Run the game loop running = True while running: # Handle user input for event in pygame.event.get(): if event.type == pygame.QUIT: running = False elif event.type == pygame.KEYDOWN: if event.key == pygame.K_LEFT: vx = -1 elif event.key == pygame.K_RIGHT: vx = 1 elif event.key == pygame.K_UP: vy = -1 elif event.key == pygame.K_DOWN: vy = 1 elif event.type == pygame.KEYUP: vx = 0 vy = 0 # Update the ball's position x += vx y += vy # Check if the ball is out of bounds and reverse its velocity if necessary if x - 20 < 0 or x + 20 > 640: vx = -vx if y - 20 < 0 or y + 20 > 480: vy = -vy # Draw the ball and update the display screen.fill((0, 0, 0)) pygame.draw.circle(screen, (255, 255, 255), (x, y), 20) pygame.display.flip() clock.tick(60) # Quit Pygame when the game loop is finished pygame.quit()
Next
This code adds some if statements that check if the ball’s x or y position is out of bounds (less than 0 or greater than the screen width/height). If the ball is out of bounds, its velocity is reversed by multiplying it by -1. This causes the ball to bounce off the edges of the screen.


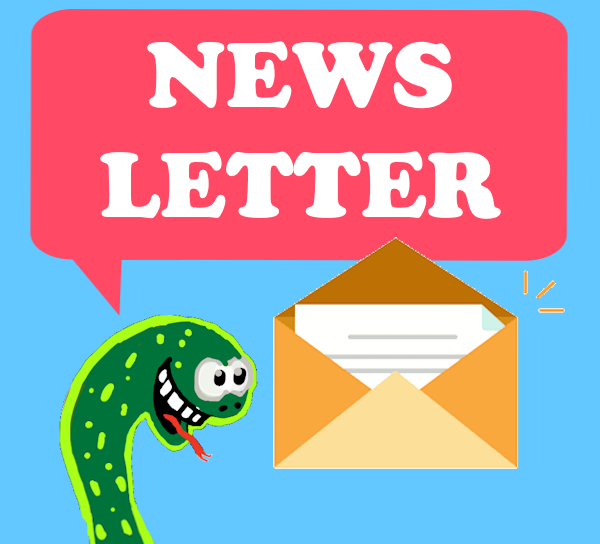


Twitter: @pythonprogrammi - python_pygame
Videos
Speech recognition gamePygame's Platform Game
