
Here is another example of use of tkinter that you can find here among other examples:
https://github.com/formazione/tkinter_tutorial.git
The raw code of this specific file is here:
https://github.com/formazione/tkinter_tutorial/blob/master/filebrowser.py
This time we look at the Listbox widget and we make a simple example about getting the text of the item selected in the listbox. In the code you can see how to insert values and other basic stuffs that will tell you most of the thing you need to use this widget. Cool, right? This is one of the most useful widget together with labels and button (and Text too). Let’s go.
To get the text of the selected item in a Listbox widget in Tkinter, you can use the .get() method with the index of the selected item. Here’s how you can do it:
import tkinter as tk class MyApplication: def __init__(self, root): self.root = root self.frame = tk.Frame(self.root) self.frame.pack() self.list_tk = tk.Listbox(self.frame) self.list_tk.pack(side="left", anchor="n") self.list_tk.insert("end", "Item 1") self.list_tk.insert("end", "Item 2") self.list_tk.insert("end", "Item 3") self.get_selected_button = tk.Button(self.frame, text="Get Selected", command=self.get_selected_item) self.get_selected_button.pack() def get_selected_item(self): selected_indices = self.list_tk.curselection() if selected_indices: selected_index = selected_indices[0] selected_item = self.list_tk.get(selected_index) print("Selected item:", selected_item) if __name__ == "__main__": root = tk.Tk() app = MyApplication(root) root.mainloop()

Here you can see the output.
In this example, the get_selected_item method is called when the “Get Selected” button is clicked. Inside this method:
The curselection() method of the Listbox returns a tuple containing the indices of the selected items. We’re assuming you’re working with a single selection, so we’re using selected_indices[0] to get the index of the first selected item.
The .get() method is used to retrieve the text of the selected item using the selected index.
The selected item’s text is printed to the console.
Remember that the curselection() method returns a tuple of selected indices, even if you’re working with a single selection listbox, hence we’re using selected_indices[0] to get the actual index value.
Let’s make a slightly different code
This time we are gonna use the bind method applied to the listbox, instead of using a button.
This script shows the py files in a directory.
from glob import glob import tkinter as tk class Show: def __init__(self): self.files = glob("*.py") def __call__(self): self.window() def window(self): self.root = tk.Tk() self.showfilestk() self.root.mainloop() def showfilestk(self): self.frame = tk.Frame(self.root) self.frame.pack() self.list_tk = tk.Listbox(self.frame) self.list_tk.pack( side="left", anchor="n", fill="both") # self.list_tk.bind("<Button>", self.show_doc) self.list_tk.bind("<<ListboxSelect>>", self.show_doc) # self.list_tk.bind("<ButtonRelease-1>", self.get_selected_item) self.text = tk.Text(self.frame) self.text.pack(side="left") for f in self.files: self.list_tk.insert(tk.END, f) self.list_tk.pack() def show_doc(self, event=' '): selected_indices = self.list_tk.curselection() selected_index = selected_indices[0] selected_item = self.list_tk.get(selected_index) print("Selected item:", selected_item) show = Show() show()

Show the item selected into the text widget
Let’s add the 2 final lines to this method to do show the item name into the text widget, instead of just printing it into the terminal.
def show_doc(self, event=' '): selected_indices = self.list_tk.curselection() selected_index = selected_indices[0] selected_item = self.list_tk.get(selected_index) print("Selected item:", selected_item) self.text.delete("0.0", tk.END) self.text.insert("0.0", selected_item)
Show the content of the files
Now, with some more changes we can have the content of the files selected into the text widget.
# filebrowser.py from glob import glob import tkinter as tk class Show: def __init__(self): self.files = glob("*.py") def __call__(self): self.window() def window(self): self.root = tk.Tk() self.showfilestk() self.root.mainloop() def showfilestk(self): self.frame = tk.Frame(self.root) self.frame.pack() self.list_tk = tk.Listbox(self.frame) self.list_tk.pack( side="left", anchor="n", fill="both") # self.list_tk.bind("<Button>", self.show_doc) self.list_tk.bind("<<ListboxSelect>>", self.show_doc) # self.list_tk.bind("<ButtonRelease-1>", self.get_selected_item) self.text = tk.Text(self.frame) self.text.pack(side="left") for f in self.files: self.list_tk.insert(tk.END, f) self.list_tk.pack() def intext(self, text): ''' insert the name of the text passed as argument ''' self.text.insert(tk.END, text) def text_delete(self): ''' delete everything in text widget ''' self.text.delete("0.0", tk.END) def intext_filecontent(self, filename): ''' open file name (item selected) and shows content ''' with open(filename) as file: self.intext(file.read()) def show_doc(self, event=' '): ''' get the item name and stores it in selected_item to show info about it (file.py)''' selected_indices = self.list_tk.curselection() selected_index = selected_indices[0] selected_item = self.list_tk.get(selected_index) print("Selected item:", selected_item) self.text_delete() self.intext_filecontent(selected_item) # with open(selected_item) as file: show = Show() show()


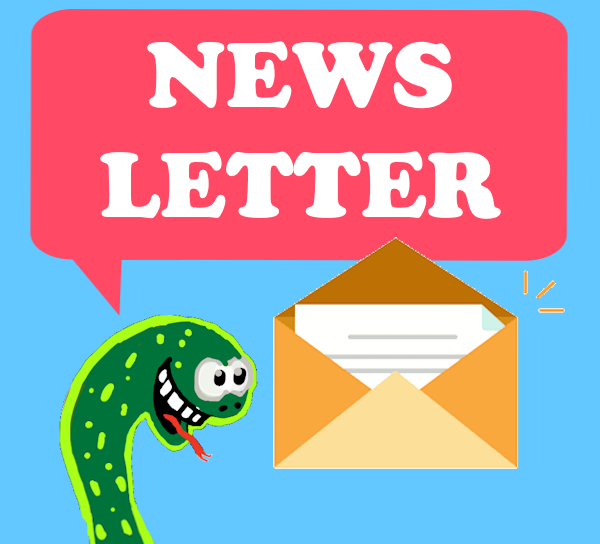


Twitter: @pythonprogrammi - python_pygame
Videos
Speech recognition gamePygame's Platform Game
