If you want to create a GUI for the sites that you prefer or to open your files on the pc, so that you remember them easily, you can use this tkinter app.
You will write the address for the buttons in a txt file called sites.txt, like the one you see down here.

You have the icons in the folder icons.

They must be png files. You put their name after a sign “#” in the same line of the addres as you saw in the image of the file sites.txt.
So it is pretty easy to add or change addresses and icons.

import tkinter as tk import tkinter.ttk as ttk from glob import glob import os root = tk.Tk() root.title("My launcher") class Button: row = 0 column = 0 def __init__(self, text, func, arg, image=""): if image!= "": image = tk.PhotoImage(file=image) root.rowconfigure(Button.row, weight=1, minsize=40) root.columnconfigure(Button.column, weight=1, minsize=40) self.button = ttk.Button( root, text=text, image=image, compound=tk.LEFT, command=lambda: func(arg)) self.button.grid(row=Button.row, column=Button.column, sticky="nsew", padx=0) self.button.image = image if Button.column < 5: Button.column += 1 else: Button.column = 0 Button.row += 1 sites = glob("*.site") print(sites) if sites != []: with open(sites[0]) as file: file = file.readlines() for line in file: x = line.strip() x = [f.strip() for f in x.split("#")] title, address, image = x Button(title, os.startfile, address, image="icons/" + image) root.mainloop()
The code


https://github.com/formazione/tkinter_tutorial/blob/master/py_site_launcher/pylauncher.py

Another example of links converted in buttons in tkinter.
It allows 5 buttons for row, then it adds a new row. You have to choose the size of the image to make it fit with the screen or put a fixed weight and height, if you do not want to spend time making the png of the exact size.
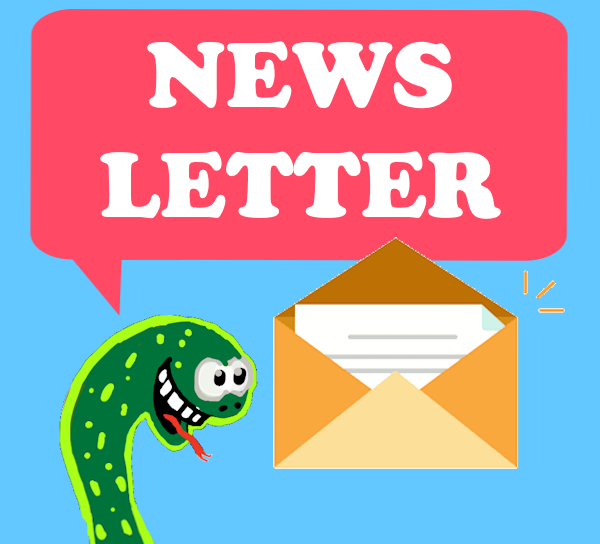


Twitter: @pythonprogrammi - python_pygame
Videos
Speech recognition gamePygame's Platform Game
