In this period we are looking at windows in tkinter
I made some code about buttons in pygame, some of which I modified from other coders code:
Button widget in Pygame updated
Now, we may think… what if we use the widgets of tkinter for pygame? Well, we cannot embed them directly into a pygame window (for what I know at least) but we can open windows of tkinter with widgets from a pygame window, for example with a basic button (it’s not as nice as the one of the previous link, I advice you, but it’s just to make the example very simple).
So here is the final result:

The code
# the repository is here: https://github.com/formazione/tkinter_tutorial.git # GiovanniPython on YT # @pythonprogrammi on X import tkinter as tk from tkinter import messagebox import pygame class Window: def __init__(self): root = tk.Tk() root.title("MAIN WINDOW") hello = tk.Toplevel(root) hello.title("Hello") hello.but1 = tk.Button(hello, text="Hello Button", command= lambda : messagebox.showinfo("Hello and attention", "A button in a secundary window has been pressed abruptely!") ) hello.but1.pack() root.mainloop() pygame.init() screen = pygame.display.set_mode((800,600)) clock = pygame.time.Clock() rect = pygame.Rect(100,100,100,50) font = pygame.font.SysFont("Arial", 20) click = font.render("Click me", 1, (0,0,0)) while True: for event in pygame.event.get(): if event.type == pygame.QUIT: pygame.quit() sys.exit() if event.type == pygame.MOUSEBUTTONUP: if rect.collidepoint(pygame.mouse.get_pos()): Window() pygame.draw.rect(screen, (255, 255,255), rect) screen.blit(click, rect) pygame.display.flip() clock.tick(60)
The repository
https://github.com/formazione/tkinter_tutorial.git
The name of the script is tkinter_pygame.py
The code of the tkinter windows is also, without the pygame code, in toplevel_simple.py
If want, we can make a button class to use it as a constructor
# the repository is here: https://github.com/formazione/tkinter_tutorial.git # GiovanniPython on YT # @pythonprogrammi on X import tkinter as tk from tkinter import messagebox import pygame class Window: def __init__(self): root = tk.Tk() root.title("MAIN WINDOW") hello = tk.Toplevel(root) hello.title("Hello") hello.but1 = tk.Button(hello, text="Hello Button", command= lambda : messagebox.showinfo("Hello and attention", "A button in a secundary window has been pressed abruptely!") ) hello.but1.pack() root.mainloop() pygame.init() class Button: def __init__(self,text,pos,command): self.text = text self.command = command self.font = pygame.font.SysFont("Arial", 20) self.text = self.font.render(text, 1, (0,0,0)) self.w, self.h = self.text.get_size() self.x, self.y = pos self.rect = pygame.Rect(self.x, self.x, self.w, self.h) def change_text(self, newtext): self.text = font.render(newtext, 1, (0,0,0)) def blit(self): pygame.draw.rect(screen, (255, 255,255), self.rect) screen.blit(self.text, (self.x, self.y)) screen = pygame.display.set_mode((800,600)) clock = pygame.time.Clock() button = Button("Click me", (100,100), None) while True: for event in pygame.event.get(): if event.type == pygame.QUIT: pygame.quit() sys.exit() if event.type == pygame.MOUSEBUTTONUP: if button.rect.collidepoint(pygame.mouse.get_pos()): Window() button.blit() pygame.display.flip() clock.tick(60)

Now the size of the button is better defined, because it is generated on the base of the size of the text, so that we do not have to manually input the width and the height as it’s calculated by the get_size method of the Surface object that is generated by the render method of SysFont.
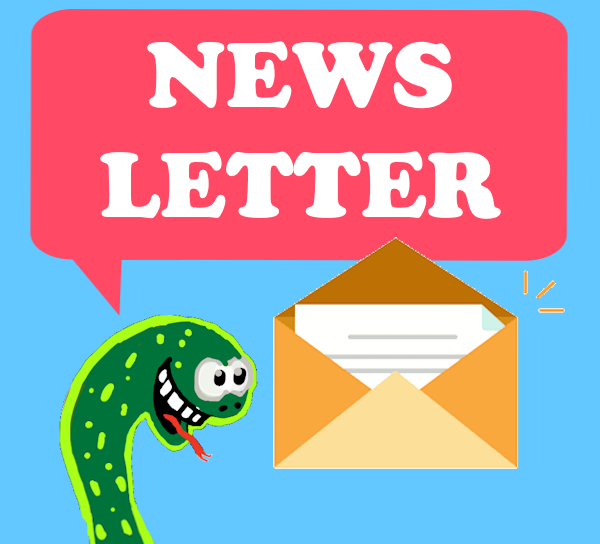


Twitter: @pythonprogrammi - python_pygame
Videos
Speech recognition gamePygame's Platform Game
