Binding
Binding is what you do to link an action to the user to a code that makes something. In this example (from Effbot, with some changes), we see how to print the coordinates of the mouse pointer when the user clicks on the Frame.
-
import tkinter as tk
-
root = tk.Tk()
-
-
def clicked(event):
-
label[‘text’] = event.x, event.y
-
frame = tk.Frame(root, width=100, height=100)
-
frame[“bg”] = “yellow”
-
frame.bind(“<Button-1>”, clicked)
-
label = tk.Label(root)
-
root.mainloop()
A little game with Frame and binding
In the following code I made some changes to create a little game where you have to find a secret spot. When you are close the Frame becomes orange, when you hit the spot it becomes red.
-
import tkinter as tk
-
import random
-
root = tk.Tk()
-
def clicked(event):
-
label[‘text’] = event.x, event.y
-
label[‘text’] = “YOU WIN!”
-
label[‘bg’] = “yellow”
-
frame[‘bg’] = “red”
-
label[‘text’] = “You’re close”
-
label[‘bg’] = “yellow”
-
frame[‘bg’] = “orange”
-
else:
-
frame[‘bg’] = “yellow”
-
x = random.randint(1, 99)
-
y = random.randint(1, 99)
-
frame = tk.Frame(root, width=100, height=100)
-
frame[“bg”] = “yellow”
-
frame.bind(“<Button-1>”, clicked)
-
label = tk.Label(root)
The game in action
In this video you can see what’s the game like. It’s a very basic example to test the use of binding.
Tkinter test for students
Tkinter articles
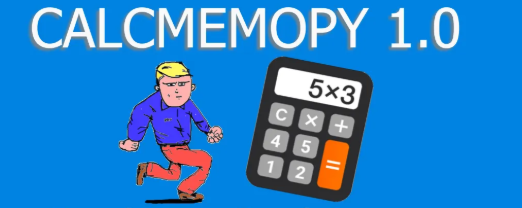