Insert items in the code
import tkinter as tk win = tk.Tk() lb = tk.Listbox(win) lb.pack() lb.insert(0, "First item") lb.insert(0, "Second item") win.mainloop()
Delete Items
import tkinter as tk win = tk.Tk() lb = tk.Listbox(win) lb.pack() lb.insert(0, "First item") lb.insert(0, "Second item") lb.delete(tk.ACTIVE) # delete the on on top lb.delete(0) # delete the one on top win.mainloop()
Select more items at once
You may be wanting to select more items at once. You must add the selectmode argument to do that, like in the code below.
import tkinter as tk win = tk.Tk() lb = tk.Listbox(win, selectmode=tk.EXTENDED) lb.pack() lb.insert(0, "First item") lb.insert(0, "Second item") win.mainloop()
Listbox: insert an item with a widget
listbox.insert(tk.END, content.get())
To insert the value in a listbox the first argument is where to start (from the last character in the listbox) and the second is the string with the item. In this case the string was taken from the content of the entry widget.
Delete all the items
listbox.delete(0, tk.END)
Delete selected item
listbox.delete(tk.ANCHOR)
Whole code
import tkinter as tk win = tk.Tk() def additem(): listbox.insert(tk.END, content.get()) def deleteAll(): listbox.delete(0, tk.END) def deleteselected(): listbox.delete(tk.ANCHOR) content = tk.StringVar() entry = tk.Entry(win, textvariable=content) entry.pack() button = tk.Button(win, text="Add Item", command=additem) button.pack() button2 = tk.Button(win, text="Delete list", command=deleteAll) button2.pack() button3 = tk.Button(win, text="Delete selected", command=deleteselected) button3.pack() listbox = tk.Listbox(win) listbox.pack() win.mainloop()
Get the selected item
import tkinter as tk # WINDOW CREATION win = tk.Tk() geo = win.geometry geo("400x400+400+400") # ACTION TO PERFORM WHEN SELECTED def go(): cs = lb.curselection()[0] label['text'] = lb.get(cs) # THE LISTBOX AND THE BINDING lb = tk.Listbox(win) lb.bind("<<ListboxSelect>>", lambda x: go()) lb.pack() # THE ITEMS INSERTED WITH A LOOP items = ["first", "second", "third"] for item in items: lb.insert(tk.END, item) # THE LABEL WHERE APPEARS THE SELECTED ITEM NAME label = tk.Label(win) label.pack() win.mainloop()
Tkinter test for students
Tkinter articles
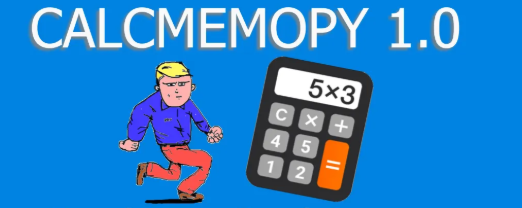