
Hi, I thought to collect some interesting templates for tkinter graphic user interfaces, so that you can soon build the ones you need without too much effort, because you can easily adapt them to you needs.
Let’s start.
Tkinter is the de facto standard GUI (Graphical User Interface) package in Python. It is a thin object-oriented layer on top of Tcl/Tk. Tkinter is not the only GUI programming toolkit for Python, but it is the most commonly used one.
Tkinter is included with standard Python distributions, so you don’t need to install anything extra to use it. To create a Tkinter GUI, you first need to import the tkinter module. Then, you can create a Tkinter window and add widgets to it. Widgets are the individual components of a GUI, such as buttons, labels, and text boxes.
Here is an example of a simple Tkinter GUI:
import tkinter def clicked(): print("You clicked the button!") window = tkinter.Tk() button = tkinter.Button(window, text="Click Me!", command=clicked) button.pack() window.mainloop()
This code creates a Tkinter window with a button in it. When the button is clicked, the clicked() function is called, which prints a message to the console.
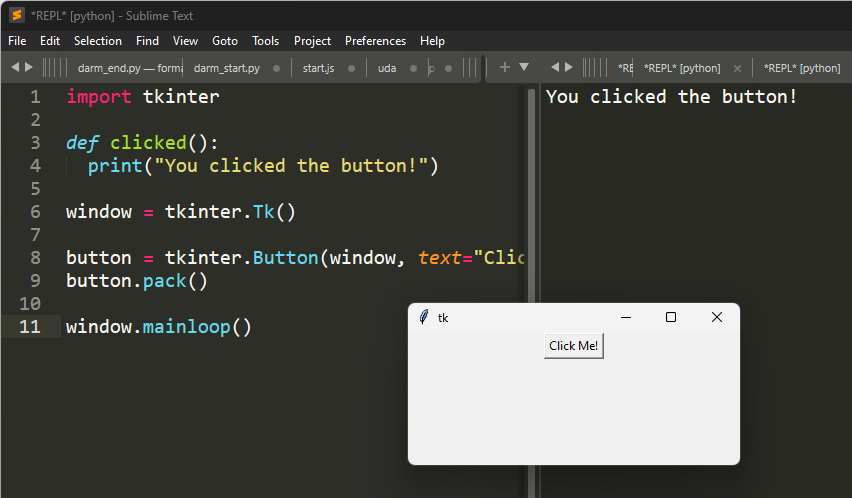
This is the simplest GUI you can do with tkinter, as you can see it’s really easy to create one. You can customize the widgets like the button, but we will see it later. I love it cause it’s so simple, don’t have to install anything cause it’s built in python installation (maybe on the MacOs you have to install it).
Index
Open file dialog
- Open File Dialog

With this one you will be able a file dialog in Windows and you will see the name of the file you selected in a message box.
Importing modules
The first few lines of code import the necessary modules:
import tkinter as tk from tkinter import ttk from tkinter import filedialog as fd from tkinter.messagebox import showinfo
The tkinter module is the main Tkinter module. The ttk module provides more modern widgets that are not part of the standard Tkinter library. The filedialog module provides functions for opening and saving files. The messagebox module provides functions for displaying messages to the user.
The root window
The next few lines of code create the root window:
# create the root window root = tk.Tk() root.title('Tkinter Open File Dialog') root.resizable(False, False) root.geometry('300x150')
The select_file() function is called when the user clicks the “Open a File” button. The function first defines a list of file types that the user can select. In this case, the user can select text files or all files.
The function then calls the askopenfilename() function from the filedialog module. The askopenfilename() function displays a dialog box that allows the user to select a file. The function returns the name of the selected file.
The function then calls the showinfo() function from the messagebox module. The showinfo() function displays a message box with the selected file name.
The button to open the file
The next line of code creates the “Open a File” button:
# open button open_button = ttk.Button( root, text='Open a File', command=select_file )

The open_button variable refers to the “Open a File” button. The ttk.Button() constructor creates a new button. The text argument sets the text of the button. The command argument sets the function that is called when the button is clicked.
When the user clicks the “Open a File” button, the select_file() function is called. The select_file() function displays a dialog box that allows the user to select a file. The user can select a text file or any other file. When the user selects a file, the name of the file is returned to the select_file() function. The select_file() function then displays a message box with the file name.
The application continues to run until the user closes the window.
The whole code with a label
import tkinter as tk from tkinter import filedialog def open_file_dialog(): file_path = filedialog.askopenfilename(filetypes=[("Text Files", "*.txt"), ("All Files", "*.*")]) if file_path: label.config(text=f"Selected file: {file_path}") # Create the main window window = tk.Tk() window.title("File Dialog Example") # Button to open file dialog button = tk.Button(window, text="Open File", command=open_file_dialog) button.pack(pady=10) # Label to display the selected file name label = tk.Label(window, text="Selected file: ") label.pack() # Start the main event loop window.mainloop()

Entry and Text
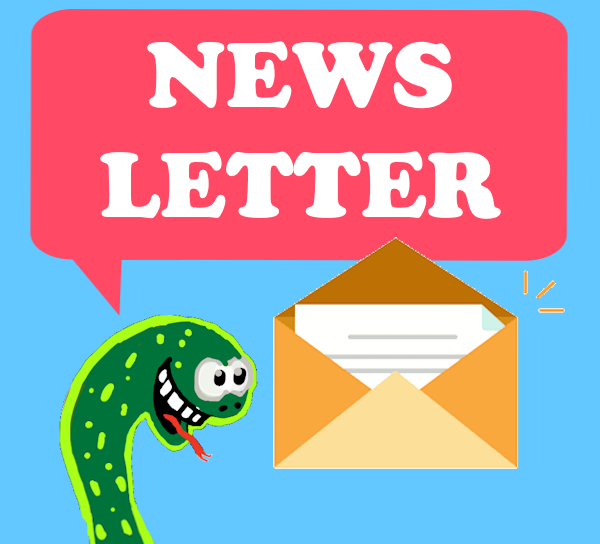


Twitter: @pythonprogrammi - python_pygame
Videos
Speech recognition gamePygame's Platform Game
